python flask 开发手机端商品保质期检查
时间: 2023-12-05 13:01:53 浏览: 32
Python Flask是一个轻量级的Python web框架,适用于开发各种类型的网站和应用程序。在进行手机端商品保质期检查的开发中,我们可以使用Python Flask来实现以下功能:
1. 用户注册和登录:首先,我们可以通过Python Flask来创建用户注册和登录功能,使用户能够创建自己的账户并登录系统。
2. 商品信息录入:用户登录后,可以录入商品的相关信息,如商品名称、生产日期、保质期等。
3. 保质期检查:在录入商品信息后,系统将自动计算出商品的过期日期,并提醒用户在过期日期前进行检查。可以通过Python Flask中的定时任务来实现自动提醒的功能。
4. 商品信息查询:用户可以通过手机端浏览已录入的商品信息,并查看每个商品的保质期情况。
5. 提醒功能:系统可以通过手机端向用户发送提醒消息,提醒用户对即将过期的商品进行检查或处理。
6. 数据统计和分析:系统可以根据录入的商品信息进行数据统计和分析,如某一时间段内过期商品的数量、过期率等。
7. 数据备份和恢复:为了保障数据的安全性,可以使用Python Flask来实现数据的备份和恢复功能,以防止数据丢失或损坏。
通过使用Python Flask开发手机端商品保质期检查,可以方便用户随时随地进行商品信息管理和保质期检查,提高了商品管理的效率和准确性。
相关问题
python flask 开发框架 pdf
Python Flask 是一个轻量级的 Web 开发框架,它提供了快速搭建 Web 应用的能力。在 Flask 中,我们可以使用第三方库来生成 PDF 文件,比如使用 ReportLab 或者 WeasyPrint。这些库可以帮助我们在 Flask 应用中生成高质量的 PDF 文件。
首先,我们需要在 Flask 项目中安装相应的生成 PDF 的库。比如,如果选择使用 ReportLab,我们可以使用 pip 命令安装:
```
pip install reportlab
```
然后,在 Flask 的视图函数中,我们可以使用这些库来生成 PDF。比如,我们可以使用 ReportLab 来创建一个 PDF 文件并将其返回给客户端:
```python
from reportlab.pdfgen import canvas
from flask import make_response
from io import BytesIO
@app.route('/generate_pdf', methods=['GET'])
def generate_pdf():
buffer = BytesIO()
pdf = canvas.Canvas(buffer)
pdf.drawString(100, 100, "Hello, World!")
pdf.showPage()
pdf.save()
buffer.seek(0)
response = make_response(buffer.getvalue())
response.headers['Content-Type'] = 'application/pdf'
response.headers['Content-Disposition'] = 'attachment; filename=hello.pdf'
return response
```
在这个例子中,我们创建了一个视图函数来生成 PDF 文件。首先,我们创建了一个 BytesIO 缓冲区,并使用 ReportLab 创建了一个 PDF 文件。然后,我们使用 Flask 的 make_response 函数将 PDF 文件返回给客户端。
总之,通过使用第三方库,我们可以在 Python Flask 中快速开发生成 PDF 的功能,满足项目的需求。
python flask开发网页后端
如果你想使用 Flask 开发网页后端,可以按照以下步骤进行:
1. 安装 Flask
你可以通过 pip 工具在命令行中输入以下命令安装 Flask:
```
pip install Flask
```
2. 创建 Flask 应用
在你的项目目录下,创建一个名为 `app.py` 的 Python 文件。然后,在文件中导入 Flask 并创建一个应用:
```python
from flask import Flask
app = Flask(__name__)
```
3. 创建路由
Flask 的路由定义了不同的 URL 如何被处理。在 `app.py` 文件中,你可以使用 `@app.route` 装饰器来定义路由。例如,以下代码定义了一个处理 `/hello` URL 的路由:
```python
@app.route('/hello')
def hello():
return 'Hello, World!'
```
4. 运行应用
可以在命令行中输入以下命令来运行应用:
```
export FLASK_APP=app.py
flask run
```
这将启动 Flask 服务器,并在默认端口上监听请求。可以在浏览器中输入 `http://localhost:5000/hello` 地址,查看输出结果。
以上是 Flask 开发网页后端的基本步骤,你可以根据自己的需求进一步开发和完善。
相关推荐
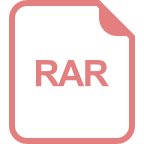
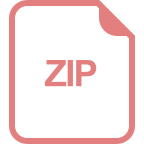











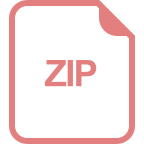