c# 读取文件返回二进制流,给出范例
时间: 2024-11-12 18:43:28 浏览: 59
在C#中,要读取文件并将其内容作为二进制流返回,你可以使用File.ReadAllBytes
方法。这个方法接收文件路径作为参数,然后读取整个文件的内容并作为一个字节数组(二进制流)返回。以下是一个简单的示例:
using System;
using System.IO;
public byte[] ReadBinaryFile(string filePath)
{
if (!File.Exists(filePath))
{
throw new FileNotFoundException("The file does not exist.", filePath);
}
try
{
return File.ReadAllBytes(filePath);
}
catch (IOException e)
{
throw new IOError("An error occurred while reading the file: " + e.Message);
}
}
在这个例子中,首先检查文件是否存在,然后尝试读取文件。如果在读取过程中发生异常,如IO错误,会捕获异常并抛出。
相关问题
c# 读取word文件返回二进制流,给出范例
在 C# 中,你可以使用 DocumentFormat.OpenXml
模块来读取 Word 文档(.docx
格式)并将其转换为二进制流。以下是一个简单的示例:
using System.IO;
using DocumentFormat.OpenXml.Packaging;
public byte[] ReadWordDocumentToBinary(string filePath)
{
// 创建一个 Stream 对象来存储二进制数据
using (var memoryStream = new MemoryStream())
{
// 加载 Word 文档
using (var document = WordprocessingDocument.Open(filePath, true))
{
// 获取主文档的部分
var mainPart = document.MainDocumentPart;
// 将文档内容写入内存流
mainPart.Document.SaveAs(memoryStream);
}
// 返回内存流中的二进制数据
return memoryStream.ToArray();
}
}
在这个例子中,我们首先打开 Word 文档,然后获取主文档的部分。接着,我们将整个文档保存到一个内存流中,最后将内存流的内容转换为字节数组。
c# 下载https开头的网址文件,给出具体范例
在C#中,你可以使用HttpClient
类以及WebClient
类来下载HTTP开头的网址文件。这里我会展示一个使用HttpClient
的例子,因为它更现代且功能更强大:
using System;
using System.IO;
using System.Net.Http;
class Program
{
static async Task Main()
{
// 定义你要下载的URL
string url = "https://example.com/file.pdf";
try
{
// 创建HttpClient实例
using HttpClient client = new HttpClient();
// 发送GET请求并获取响应内容
HttpResponseMessage response = await client.GetAsync(url);
// 检查请求是否成功(状态码200表示成功)
if (response.IsSuccessStatusCode)
{
// 设置响应内容类型为二进制流,以便读取文件数据
response.Content.Headers.ContentType = new MediaTypeHeaderValue("application/octet-stream");
// 获取响应内容为字节流
byte[] bytes = await response.Content.ReadAsByteArrayAsync();
// 将字节数组保存到本地文件
string filePath = "local_file.pdf"; // 替换为你需要保存的本地路径
File.WriteAllBytes(filePath, bytes);
Console.WriteLine($"文件已下载至: {filePath}");
}
else
{
Console.WriteLine($"请求失败,状态码: {response.StatusCode}");
}
}
catch (Exception ex)
{
Console.WriteLine($"下载过程中发生错误: {ex.Message}");
}
}
}
这个示例中,我们首先创建了一个HttpClient
,然后发送一个GET请求到指定的URL。如果服务器返回的状态码是200(成功),我们就将响应的内容作为字节数组保存到本地。
相关推荐






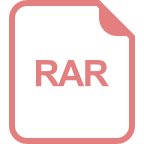
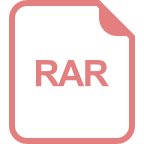
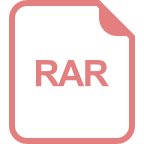

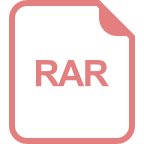
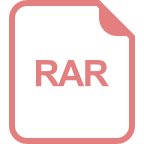
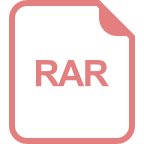



