用springboot构建一个学生信息增删改查框架
时间: 2024-12-19 18:23:12 浏览: 7
在Spring Boot中构建一个学生信息管理框架通常涉及以下几个步骤:
1. **项目初始化**:
创建一个新的Spring Boot项目,可以选择Maven或Gradle作为构建工具。
2. **创建数据模型**:
定义`Student`实体类,包含属性如学号、姓名、性别、出生日期等,并添加相应的getter和setter方法。
```java
public class Student {
private Long id;
private String name;
// ...其他属性和构造函数
}
```
3. **数据库配置**:
配置JPA或MyBatis等持久层技术,连接到数据库并映射`Student`实体到数据库表。
4. **Repository接口**:
创建`StudentRepository`接口,该接口通常由Spring Data JPA自动生成,用于CRUD操作。
```java
public interface StudentRepository extends JpaRepository<Student, Long> {
List<Student> findAll();
// ...其他方法如findById(), save(), deleteById()
}
```
5. **Service层**:
设计`StudentService`类,注入`StudentRepository`,实现对学生信息的增删改查功能。
6. **Controller层**:
创建RESTful API控制器,例如`StudentController`,处理HTTP请求,通过`@Autowired`注入`StudentService`来调用业务逻辑。
```java
@RestController
@RequestMapping("/api/students")
public class StudentController {
@Autowired
private StudentService studentService;
@PostMapping
public ResponseEntity<?> create(@Validated Student student) {
// 实现创建操作...
}
@GetMapping("/{id}")
public ResponseEntity<?> read(@PathVariable Long id) {
// 实现读取操作...
}
// 对应于update和delete方法
}
```
7. **异常处理**:
使用Spring MVC的`@ExceptionHandler`处理可能出现的异常,提供友好的错误提示给前端。
阅读全文
相关推荐
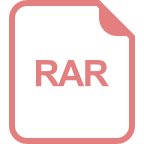
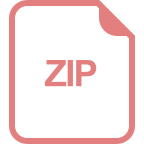
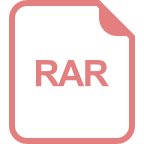
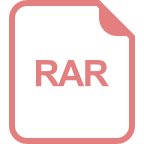
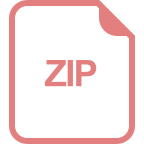
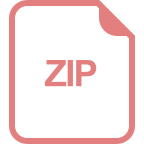
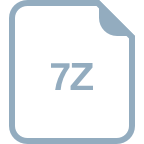
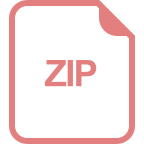
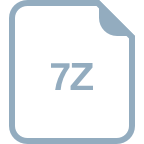
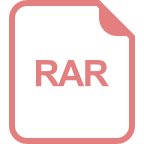
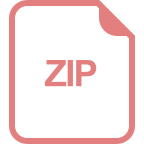
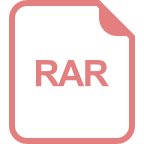
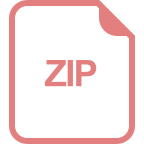
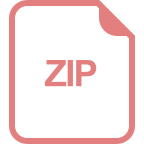
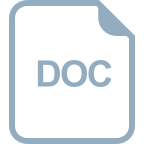
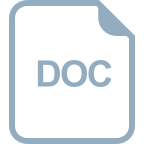
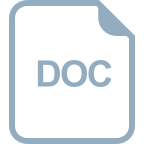
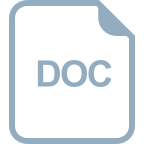
