android studio生成四个田字格
时间: 2023-11-16 12:00:16 浏览: 159
要生成四个田字格,可以使用Android Studio进行开发,具体实现方式如下:
```java
//Java代码
public class MainActivity extends AppCompatActivity {
private int[][] block = new int[4][4]; //定义一个4*4的数组,用于存储方块
private int[][][] blocks = new int[][][]{ //定义七种不同的方块
{
{0, 0, 0, 0},
{0, 0, 0, 0},
{0, 1, 1, 0},
{0, 1, 1, 0}
},
{
{0, 0, 0, 0},
{0, 0, 0, 0},
{0, 0, 1, 0},
{0, 1, 1, 1}
},
{
{0, 0, 0, 0},
{0, 0, 0, 0},
{0, 1, 1, 1},
{0, 0, 0, 1}
},
{
{0, 0, 0, 0},
{0, 0, 0, 0},
{0, 1, 1, 1},
{0, 1, 0, 0}
},
{
{0, 0, 0, 0},
{0, 0, 0, 0},
{0, 1, 1, 1},
{0, 0, 1, 0}
},
{
{0, 0, 0, 0},
{0, 0, 0, 0},
{0, 1, 1, 0},
{0, 0, 1, 1}
},
{
{0, 0, 0, 0},
{0, 0, 0, 0},
{0, 0, 1, 1},
{0, 1, 1, 0}
}
};
private int currentBlock = 0; //当前方块的编号
private int currentX = 0; //当前方块的横坐标
private int currentY = 0; //当前方块的纵坐标
private int score = 0; //得分
private boolean isOver = false; //游戏是否结束
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
initBlock(); //初始化方块
new Thread(new Runnable() { //开启一个新线程,用于控制方块的下落
@Override
public void run() {
while (!isOver) { //游戏未结束时不断循环
try {
Thread.sleep(1000); //每隔1秒钟方块下落一格
} catch (InterruptedException e) {
e.printStackTrace();
}
if (canMoveDown()) { //如果方块可以向下移动
currentY++; //纵坐标加1
} else { //否则
mergeBlock(); //将方块合并到底部的方块中
checkFullLine(); //检查是否有满行
newBlock(); //生成新的方块
if (!canMoveDown()) { //如果新的方块无法向下移动
isOver = true; //游戏结束
}
}
runOnUiThread(new Runnable() { //在UI线程中更新界面
@Override
public void run() {
updateUI(); //更新界面
}
});
}
}
}).start();
}
private void initBlock() { //初始化方块
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
block[i][j] = 0; //将方块数组中的所有元素都设为0
}
}
}
private void newBlock() { //生成新的方块
currentBlock = (int) (Math.random() * 7); //随机生成一个方块编号
currentX = 3; //将横坐标设为3
currentY = 0; //将纵坐标设为0
if (!canMoveDown()) { //如果新的方块无法向下移动
isOver = true; //游戏结束
}
}
private boolean canMoveDown() { //判断方块是否可以向下移动
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
if (blocks[currentBlock][i][j] != 0) { //如果方块的这个位置有方块
if (currentY + i == 19) { //如果方块已经到达底部
return false; //返回false
}
if (block[currentY + i + 1][currentX + j] != 0) { //如果方块下面已经有方块
return false; //返回false
}
}
}
}
return true; //否则返回true
}
private void mergeBlock() { //将方块合并到底部的方块中
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
if (blocks[currentBlock][i][j] != 0) { //如果方块的这个位置有方块
block[currentY + i][currentX + j] = currentBlock + 1; //将方块的颜色值赋给底部的方块
}
}
}
}
private void checkFullLine() { //检查是否有满行
for (int i = 19; i >= 0; i--) { //从下往上遍历每一行
boolean isFull = true; //假设这一行是满行
for (int j = 0; j < 10; j++) { //遍历这一行的每一个方块
if (block[i][j] == 0) { //如果这个方块为空
isFull = false; //这一行不是满行
break; //跳出循环
}
}
if (isFull) { //如果这一行是满行
score += 10; //得分加10分
for (int k = i; k > 0; k--) { //将这一行上面的所有行都向下移动一行
for (int j = 0; j < 10; j++) {
block[k][j] = block[k - 1][j];
}
}
i++; //重新检查这一行
}
}
}
private void updateUI() { //更新界面
TextView tvScore = findViewById(R.id.tv_score); //得分文本框
tvScore.setText("得分:" + score); //显示得分
GridLayout glBlock = findViewById(R.id.gl_block); //方块布局
glBlock.removeAllViews(); //清空方块布局
for (int i = 0; i < 20; i++) { //遍历每一行
for (int j = 0; j < 10; j++) { //遍历每一列
ImageView ivBlock = new ImageView(this); //创建一个ImageView
ivBlock.setLayoutParams(new GridLayout.LayoutParams()); //设置布局参数
ivBlock.setScaleType(ImageView.ScaleType.CENTER_INSIDE); //设置缩放类型
ivBlock.setPadding(2, 2, 2, 2); //设置内边距
switch (block[i][j]) { //根据方块的颜色值设置不同的图片
case 0:
ivBlock.setImageResource(R.drawable.block_empty);
break;
case 1:
ivBlock.setImageResource(R.drawable.block_blue);
break;
case 2:
ivBlock.setImageResource(R.drawable.block_cyan);
break;
case 3:
ivBlock.setImageResource(R.drawable.block_green);
break;
case 4:
ivBlock.setImageResource(R.drawable.block_magenta);
break;
case 5:
ivBlock.setImageResource(R.drawable.block_orange);
break;
case 6:
ivBlock.setImageResource(R.drawable.block_red);
break;
case 7:
ivBlock.setImageResource(R.drawable.block_yellow);
break;
}
glBlock.addView(ivBlock); //将ImageView添加到方块布局中
}
}
}
}
```
在布局文件中添加一个GridLayout,用于显示方块:
```xml
<!--XML代码-->
<GridLayout
android:id="@+id/gl_block"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:columnCount="10"
android:rowCount="20"
android:background="#000000"
android:layout_margin="10dp"
android:padding="2dp"
android:layout_centerInParent="true">
</GridLayout>
```
然后在MainActivity中初始化方块数组、生成新的方块、开启一个新线程用于控制方块的下落、判断方块是否可以向下移动、将方块合并到底部的方块中、检查是否有满行、更新界面等操作。最后在updateUI()方法中根据方块的颜色值设置不同的图片,并将ImageView添加到方块布局中。运行程序即可生成四个田字格。
阅读全文
相关推荐
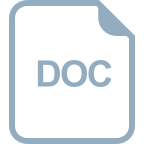
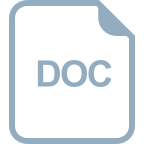
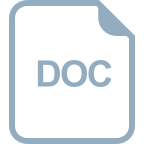
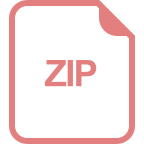
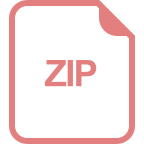
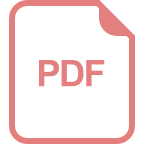
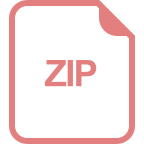
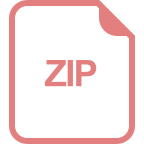
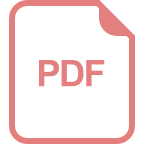
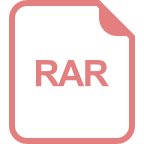
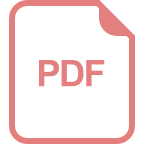
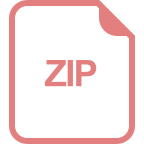
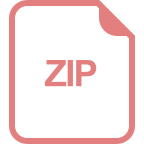
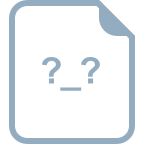
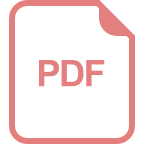
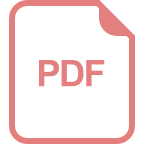