excel to mysql check data value exist
时间: 2024-12-24 07:39:58 浏览: 6
当你需要从Excel文件导入数据到MySQL数据库,并检查某个字段的值是否已存在时,可以采取以下步骤:
1. **读取Excel数据**:
使用Python的pandas库可以轻松读取Excel文件:
```python
import pandas as pd
df_excel = pd.read_excel('file.xlsx')
```
这会将Excel数据加载到一个DataFrame对象中。
2. **连接MySQL数据库**:
使用`mysql-connector-python`库建立连接:
```python
import mysql.connector
cnx = mysql.connector.connect(user='username', password='password',
host='localhost',
database='your_database')
cursor = cnx.cursor()
```
3. **遍历Excel数据并检查是否存在**:
对DataFrame中的每一行,你可以编写一条SQL查询来检查指定字段的值是否已经存在于表中:
```python
for index, row in df_excel.iterrows():
field_value = row['your_column'] # 替换 'your_column' 为你想检查的列名
query = f"SELECT COUNT(*) FROM your_table WHERE your_column = %s"
cursor.execute(query, (field_value,))
result = cursor.fetchone()
if result[0] > 0:
print(f"{field_value} already exists")
```
4. **插入新数据或处理冲突**:
根据查询结果,你可以选择插入新数据(如果不存在),或者提示用户处理冲突情况。
5. **关闭连接**:
结束操作后别忘了关闭游标和数据库连接:
```python
cursor.close()
cnx.close()
```
完成以上步骤后,你就可以在Excel导入过程中自动检查数据一致性了。
阅读全文
相关推荐
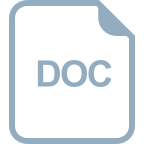
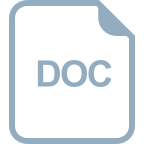
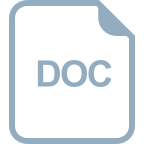
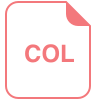
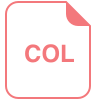
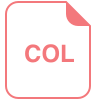
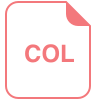
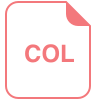
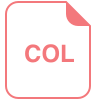
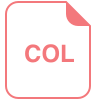
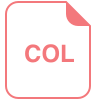
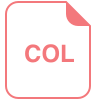
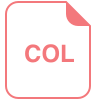
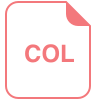
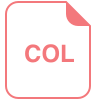
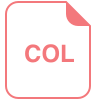
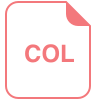

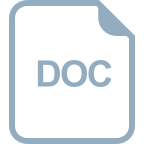