vue3ts组件相互传参
时间: 2023-11-05 16:05:30 浏览: 42
在Vue 3和TypeScript中,组件相互传参有几种方式:
1. 通过props进行父组件向子组件的传递:
- 在父组件中,使用`defineProps`定义props,并在子组件中使用`props`接收。
- 父组件使用`:属性名=属性值`的形式传递数据给子组件。
2. 通过emits进行子组件向父组件的传递:
- 在父组件中,定义一个方法,并在子组件中使用`defineEmits`定义emit。
- 子组件通过`emit(方法名, 参数)`触发父组件的方法,并传递参数。
3. 借鉴React,通过函数调用传值:
- 在父组件中定义一个方法,并将其传递给子组件。
- 子组件通过调用该方法,并传递参数进行数值的传递。
以上是Vue 3和TypeScript中实现组件相互传参的几种方式。您可以根据具体的需求选择适合的方式。
相关问题
vue3 ts子组件传参到父组件
子组件传参到父组件可以通过自定义事件和$emit方法实现。具体步骤如下:
1.在子组件中定义一个方法,用于触发自定义事件并传递参数。
```vue
<template>
<div class="child">
子组件
<button @click="handleClick">传递参数到父组件</button>
</div>
</template>
<script setup lang="ts">
import { defineEmits } from 'vue'
defineEmits(['sendData'])
const handleClick = () => {
const data = '这是子组件传递给父组件的数据'
// 触发自定义事件,并将数据作为参数传递给父组件
emit('sendData', data)
}
</script>
```
2.在父组件中通过ref获取子组件实例,并在mounted钩子函数中监听子组件的自定义事件。
```vue
<template>
<div class="parent">
父组件
<Child ref="child" @sendData="handleReceiveData"></Child>
<div>接收到的数据:{{ receivedData }}</div>
</div>
</template>
<script setup lang="ts">
import Child from './Child.vue'
import { ref, reactive } from 'vue'
const child = ref(null)
const state = reactive({
receivedData: ''
})
const handleReceiveData = (data: string) => {
// 接收子组件传递过来的数据
state.receivedData = data
}
// 在mounted钩子函数中获取子组件实例
const mounted = () => {
child.value = ref.value
}
</script>
```
3.在父组件中定义一个方法,用于接收子组件传递过来的数据。
```vue
<script setup lang="ts">
import Child from './Child.vue'
import { ref, reactive } from 'vue'
const child = ref(null)
const state = reactive({
receivedData: ''
})
const handleReceiveData = (data: string) => {
// 接收子组件传递过来的数据
state.receivedData = data
}
// 在mounted钩子函数中获取子组件实例
const mounted = () => {
child.value = ref.value
}
</script>
```
uniapp的vue3+ts组件传参怎么写
在 Uniapp 中使用 Vue3 + TypeScript 编写组件时,可以使用 `prop` 来定义组件的属性,并在组件内部使用 `props` 接收这些属性。
例如,定义一个名为 `MyComponent` 的组件,传入一个名为 `text` 的字符串属性:
```vue
<template>
<div>{{ text }}</div>
</template>
<script lang="ts">
import { defineComponent, PropType } from 'vue'
export default defineComponent({
name: 'MyComponent',
props: {
text: {
type: String as PropType<string>,
required: true
}
}
})
</script>
```
在使用 `MyComponent` 时,可以通过 `:text` 的方式传入文本:
```vue
<template>
<MyComponent :text="'Hello, world!'"/>
</template>
<script lang="ts">
import { defineComponent } from 'vue'
import MyComponent from '@/components/MyComponent.vue'
export default defineComponent({
name: 'MyPage',
components: {
MyComponent
}
})
</script>
```
注意:在 TypeScript 中,需要使用 `PropType` 来指定属性的类型,否则会编译错误。同时,由于 Uniapp 使用了跨平台的技术,因此需要在组件名称后面添加 `.vue` 后缀。
相关推荐
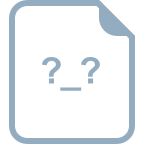










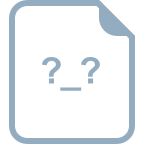
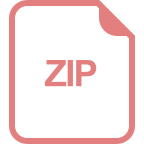