统计0和1的个数相等的数据,并以二进制形式显示输出,设计一个包括键盘输入、进制转换、数据处理、输出显示的汇编语言源程序。(c语言实现)
时间: 2024-10-28 10:15:33 浏览: 32
为了帮助您理解如何设计这样一个简单的汇编语言源程序,我们首先需要明确一点:汇编语言的具体语法和指令集因不同的架构(如x86、ARM等)而异,因此这里我会提供一个通用的大致框架,假设我们使用的是基于Intel x86体系结构的汇编语言(如NASM)。对于C语言来说,您可以直接完成这个功能,但对于初学者而言,从C语言到汇编语言的翻译会更复杂。以下是一个简化的示例:
```assembly
section .data
input db 'Enter binary string (0s and 1s only): ', 0
output db 'Equal number of zeros and ones in binary is: ', 0
format db '%d', 10
section .bss
binary resb 100
count_zeros dd 0
count_ones dd 0
section .text
global _start
_start:
; 获取用户输入
; 输入函数省略,因为这通常涉及操作系统API,不是基本汇编任务
; 但你可以想象有一个子程序读取用户输入并存储到binary变量中
; 初始化计数器
xor count_zeros, count_zeros
xor count_ones, count_ones
; 遍历输入字符串
loop_start:
lodsb ; load the next byte from binary into AL
cmp al, '0' ; compare with '0'
je zero_counter ; jump if equal to 0
cmp al, '1' ; compare with '1'
je one_counter ; jump if equal to 1
jmp continue_loop ; otherwise, skip to the next byte
zero_counter:
inc count_zeros
jmp continue_loop
one_counter:
inc count_ones
continue_loop:
cmp byte [binary], 0 ; check for end of string
jnz loop_start ; if not, continue
; 比较零和一的数量
cmp count_zeros, count_ones
je same_count ; jump if counts are equal
; 如果不等,输出错误信息
output_error:
mov eax, 4 ; sys_write syscall
mov ebx, 1 ; stdout
mov ecx, output ; error message
mov edx, format
int 0x80 ; call kernel service
; 否则,计算并输出二进制表示的次数
same_count:
sub count_zeros, count_ones ; since we're XORing these initially
add count_zeros, count_ones ; we'll get the absolute difference
add eax, count_zeros
call print_number ; a hypothetical function to print an integer in decimal
; 输出结束符
lea ebx, [output + format_len]
mov eax, 4 ; sys_write syscall
mov edx, 2 ; length of end string
int 0x80
exit:
mov eax, 1 ; sys_exit syscall
xor ebx, ebx ; exit code 0
int 0x80
print_number:
; Implement a simple routine to print an integer in decimal using printf-like syntax.
; This function is skipped here because it's beyond the scope of basic assembly.
format_len equ $ - format ; Length of format string
```
请注意,以上代码仅为示例,实际的汇编程序将依赖于具体的汇编环境和操作系统提供的系统调用。而且,`print_number`部分通常会在汇编程序中使用更底层的方式来实现,例如通过直接操作内存映射文件或寄存器。
阅读全文
相关推荐
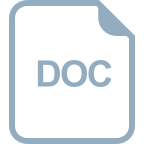
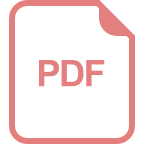
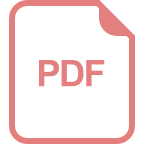


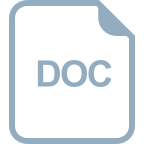
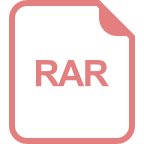
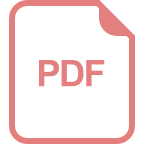
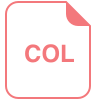
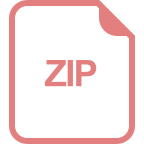
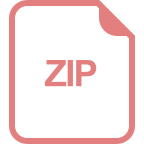
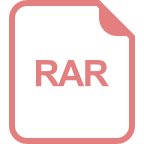
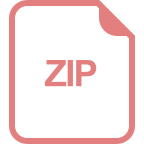
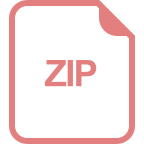
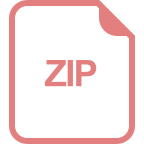