python 字符串去除特定字符
时间: 2024-09-07 18:04:43 浏览: 83
在Python中去除字符串中的特定字符可以通过多种方法实现,其中两种常用的方法是使用`str.replace()`方法和列表推导式。下面是两种方法的简单介绍:
1. 使用`str.replace()`方法:
这个方法可以在字符串中查找并替换指定的字符。如果你想要去除字符串中的特定字符,可以通过将该字符替换为空字符串来实现。
```python
original_string = "hello,world!"
modified_string = original_string.replace("!", "")
print(modified_string) # 输出: "hello,world"
```
这种方法会替换掉字符串中所有的指定字符。
2. 使用列表推导式:
列表推导式是一种更加灵活的处理方式,可以通过迭代字符串中的每个字符,并通过条件判断来决定是否将字符加入到新的字符串中。
```python
original_string = "hello,world!"
modified_string = "".join([char for char in original_string if char != "!"])
print(modified_string) # 输出: "hello,world"
```
这种方式同样可以去除字符串中的所有指定字符。
相关问题
python 字符串去除特定内容
在Python中,你可以使用`replace()`函数或者`str.remove()`方法来去除字符串中的特定内容。这两个方法都可以替换或移除指定的子字符串。
1. `replace(old, new[, count])`: 这个方法会查找所有出现的`old`子串,并将其替换为`new`。如果提供了`count`参数,那么只会替换前`count`次出现的子串。
```python
text = "Hello, World!"
new_text = text.replace(",", "")
print(new_text) # 输出: "Hello World!"
```
2. `str.remove(substring)` (适用于字符串切片): 这个方法会直接从原始字符串中删除首次出现的`substring`。如果字符串不存在,则不会做任何改变。
```python
text = "Hello, World!"
text = text[text.find(",") + 1:] # 找到逗号的位置并移除
print(text) # 输出: "World!"
```
如果你需要更复杂的正则表达式匹配和替换,可以使用`re`模块。
python字符串去掉-
在Python中,如果你想要从一个字符串中移除特定字符,如"-", 可以使用`replace()`函数或者切片操作。以下是两个例子:
1. 使用`replace()`函数:
```python
s = "example-string-with-dash"
new_string = s.replace("-", "")
print(new_string) # 输出: "examplestringwithdash"
```
2. 切片操作 (如果只关心连续的字符替换):
```python
s = "example-string-with-dash"
new_string = "".join(c for c in s if c != "-")
print(new_string) # 输出: "examplestringwithdash"
```
这两个方法都会创建一个新的字符串,原始字符串不会改变。
如果你想处理整个字符串并直接修改原字符串,可以使用`str.strip('-')`方法,它会移除字符串首尾的"-":
```python
s = "example-string-with-dash"
s = s.strip("-")
print(s) # 输出: "example-string-with"
```
阅读全文
相关推荐
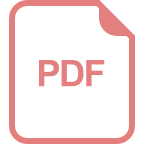
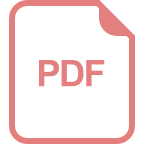
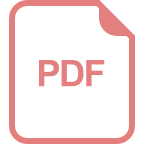

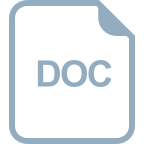
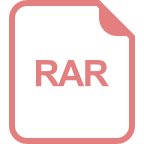
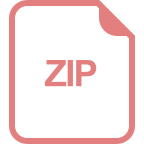
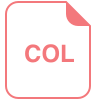
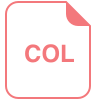
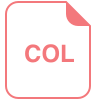
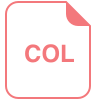
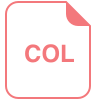




