2、定义一个链栈的类,实现以下操作:插入、删除、判断栈是否栈空、读取栈栈顶元素、析构函数。 要求实现完整程序,有必要的结果输出。 两个栈中元素值和个数自定。
时间: 2024-10-18 18:22:35 浏览: 25
```cpp
#include <iostream>
using namespace std;
// 定义节点结构体
struct Node {
int data;
Node* next;
};
class Stack {
private:
Node* top; // 栈顶指针
int size; // 栈大小
public:
// 构造函数,初始化栈为空
Stack(int capacity) {
top = nullptr;
size = capacity;
}
// 插入元素到栈顶
void push(int value) {
if (top == nullptr || size - 1 == top->next->data) {
cout << "Stack overflow!" << endl;
return;
}
Node* newNode = new Node();
newNode->data = value;
newNode->next = top;
top = newNode;
++size;
cout << "Inserted: " << value << endl;
}
// 删除栈顶元素
void pop() {
if (top == nullptr) {
cout << "Stack is empty!" << endl;
return;
}
Node* temp = top;
top = top->next;
delete temp;
--size;
cout << "Popped: " << temp->data << endl;
}
// 判断栈是否为空
bool isEmpty() const {
return top == nullptr;
}
// 读取栈顶元素,但不删除
int peek() const {
if (top == nullptr) {
cout << "Stack is empty!" << endl;
return -1; // 返回一个默认值表示栈为空
}
return top->data;
}
// 析构函数,在栈被销毁前释放内存
~Stack() {
while (!isEmpty()) {
pop();
}
cout << "Destructor called. Stack cleared." << endl;
}
};
int main() {
Stack stack(5); // 创建一个容量为5的栈
// 插入元素示例
stack.push(1);
stack.push(2);
stack.push(3);
// 读取并删除栈顶元素
cout << "Top element before pop: " << stack.peek() << endl;
stack.pop();
// 判断栈状态
cout << "Is the stack empty? " << (stack.isEmpty() ? "Yes" : "No") << endl;
return 0;
}
```
在这个例子中,我们创建了一个名为`Stack`的类,它使用链表作为底层数据结构。实现了插入、删除、查看栈顶元素以及检查栈是否为空的操作。在`main()`函数中,我们展示了如何使用这个类。
阅读全文
相关推荐
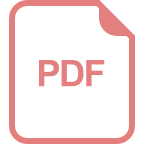
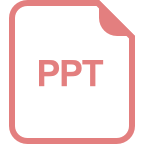
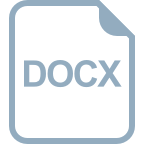















