在C#中如何导出成csv文件,并且下载地址为桌面
时间: 2024-10-15 08:22:19 浏览: 33
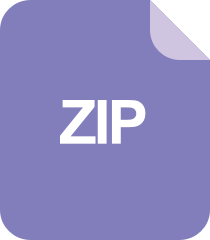
C#数据存储到本地CSV文件Demo
在C#中,你可以使用`System.IO.File`类和`HttpClient`来创建CSV文件并将其作为附件发送到用户的桌面。首先,确保已添加`System.Net.Http`命名空间。以下是一个简单的示例,展示如何完成这个任务:
```csharp
using System;
using System.IO;
using System.Net.Http;
using System.Net.Mime;
public class CsvExporter
{
public static async void ExportToCsv(string csvData, string desktopPath)
{
// 创建CSV文件路径
string filePath = Path.Combine(desktopPath, "output.csv");
// 将字符串数据写入文件
using (StreamWriter writer = new StreamWriter(filePath))
{
writer.WriteLine(csvData);
}
// 使用HttpClient创建下载链接
Uri downloadUri = new Uri($"file://{filePath}");
HttpResponseMessage response = new HttpResponseMessage(HttpStatusCode.OK)
{
Content = new ByteArrayContent(File.ReadAllBytes(filePath)),
ContentType = MediaTypeHeaderValue.Parse("text/csv"),
StatusCode = HttpStatusCode.OK,
ReasonPhrase = "Downloaded",
Headers = { { HttpHeaders.ContentDisposition, "attachment; filename=output.csv" } },
};
// 发送HTTP GET请求,模拟用户点击下载
using (HttpClient client = new HttpClient())
{
var result = await client.GetAsync(downloadUri);
if (result.IsSuccessStatusCode)
{
Console.WriteLine($"CSV file exported and downloaded at {downloadUri}");
// 这里你可以选择将下载链接显示给用户,例如在网页上或者UI组件中
// 真实应用中可能会需要考虑异常处理和用户交互逻辑
}
else
{
Console.WriteLine($"Failed to export CSV file with error: {response.ReasonPhrase}");
}
}
}
}
```
然后,你可以像这样调用这个方法:
```csharp
string csvData = "Some data in CSV format";
string desktopPath = Environment.GetFolderPath(Environment.SpecialFolder.Desktop);
CsvExporter.ExportToCsv(csvData, desktopPath);
```
阅读全文
相关推荐
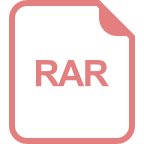
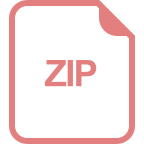

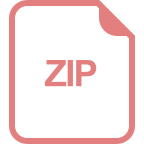
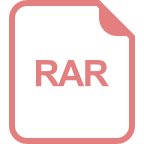
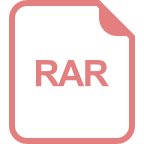
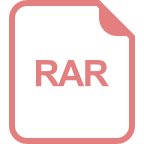
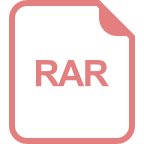
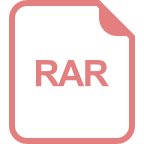
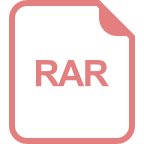
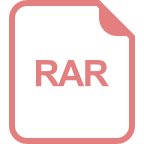
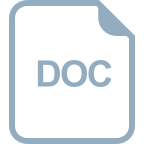
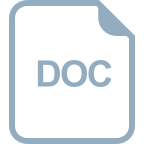
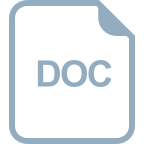
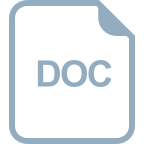

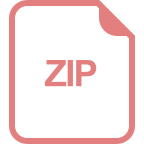
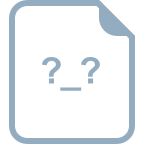