std::bind详解
时间: 2023-09-16 10:12:21 浏览: 136
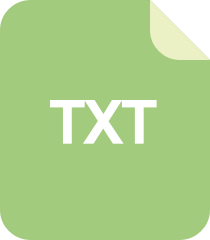
详解bind函数
std::bind是C++11引入的函数对象适配器,用于将函数对象(包括普通函数、成员函数、函数指针等)与其参数进行绑定,生成一个新的函数对象。
它的基本语法如下:
```cpp
template< class Fn, class... Args >
bind( Fn&& fn, Args&&... args );
```
其中,Fn表示要绑定的函数对象(可以是普通函数、成员函数、函数指针等),Args表示要绑定的参数。
使用std::bind可以实现参数的绑定、参数重排、占位符等功能。下面是一些示例:
1. 绑定普通函数:
```cpp
#include <iostream>
#include <functional>
void foo(int a, int b) {
std::cout << "a + b = " << a + b << std::endl;
}
int main() {
auto func = std::bind(foo, 1, 2);
func(); // 输出:a + b = 3
return 0;
}
```
2. 绑定成员函数:
```cpp
#include <iostream>
#include <functional>
class Foo {
public:
void bar(int a, int b) {
std::cout << "a + b = " << a + b << std::endl;
}
};
int main() {
Foo obj;
auto func = std::bind(&Foo::bar, &obj, 1, 2);
func(); // 输出:a + b = 3
return 0;
}
```
3. 参数重排:
```cpp
#include <iostream>
#include <functional>
void foo(int a, int b) {
std::cout << "a + b = " << a + b << std::endl;
}
int main() {
auto func = std::bind(foo, std::placeholders::_2, std::placeholders::_1);
func(2, 1); // 输出:a + b = 3
return 0;
}
```
在这个示例中,使用std::placeholders::_1和std::placeholders::_2来表示占位符,表示在调用func时,第一个参数将会被传递给foo的第二个参数,第二个参数将会被传递给foo的第一个参数。
这些仅是std::bind的一些基本用法,它还提供了更多的功能和特性,可以根据具体需求进行学习和使用。希望能对你有所帮助!
阅读全文
相关推荐
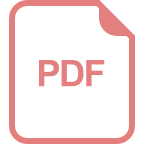
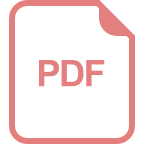



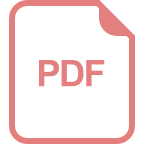
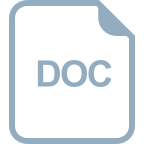


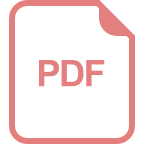
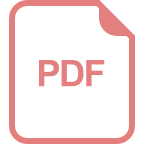
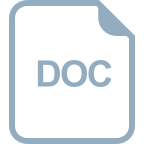
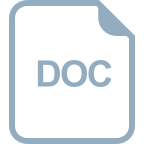
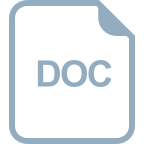
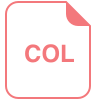
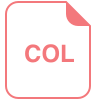