Linux Open函数
时间: 2023-12-15 20:05:58 浏览: 88
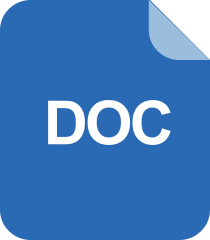
linux中open函数使用

在 Linux 系统中,open() 函数用于打开一个文件并返回一个文件描述符,以便后续的读取、写入或其他操作。它的函数原型如下:
```c
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
int open(const char *pathname, int flags);
int open(const char *pathname, int flags, mode_t mode);
```
其中,pathname 表示要打开的文件路径名,flags 表示打开文件的方式和权限,mode 是文件的访问权限。返回值为文件描述符,如果出现错误则返回 -1。
常见的 flags 标志有:
- O_RDONLY:只读打开
- O_WRONLY:只写打开
- O_RDWR:读写打开
- O_CREAT:如果文件不存在则创建
- O_TRUNC:清空文件内容
- O_APPEND:在文件末尾添加数据
常见的 mode 权限有:
- S_IRUSR:用户读权限
- S_IWUSR:用户写权限
- S_IXUSR:用户执行权限
- S_IRGRP:组读权限
- S_IWGRP:组写权限
- S_IXGRP:组执行权限
- S_IROTH:其他用户读权限
- S_IWOTH:其他用户写权限
- S_IXOTH:其他用户执行权限
示例代码:
```c
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
int main()
{
int fd = open("test.txt", O_WRONLY|O_CREAT|O_TRUNC, S_IRUSR|S_IWUSR);
if (fd == -1) {
perror("open error");
return -1;
}
write(fd, "hello world", 11);
close(fd);
return 0;
}
```
该程序打开一个名为 test.txt 的文件,如果文件不存在则创建,如果文件已经存在则清空其内容,然后写入 "hello world" 并关闭文件。
阅读全文
相关推荐
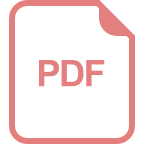
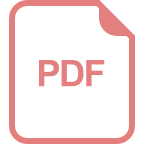
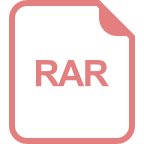
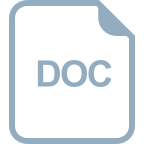
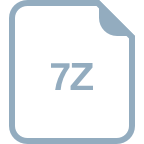
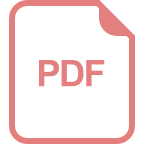
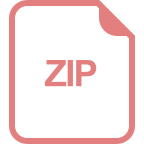
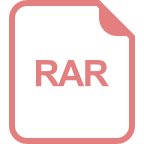
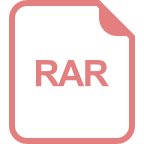
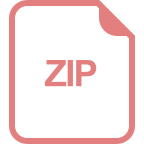
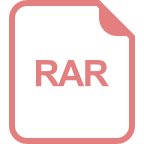
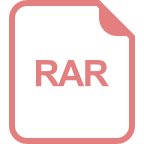
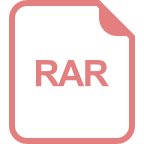

