请你根据这个题目写出问题的C++的代码裁判测试程序给出父类People、派生类Student以及测试两个类的相关C++代码。 函数接口定义: Student::Student(string id, string name, string major, int score); 在这里解释接口参数。例如:其中 N 和 D 都是用户传入的参数。 N 的值不超过int的范围; D 是[0, 9]区间内的个位数。函数须返回 N 中 D 出现的次数。 裁判测试程序样例: 在这里给出函数被调用进行测试的例子。例如: #include <iostream> #include <string> using namespace std; class Person { protected: string id; string name; public: Person(string id, string name) { this->id = id; this->name = name; } }; class Student : public Person { private: string major; int score; public: Student(string id, string name, string major, int score); friend ostream& operator <<(ostream &out, Student &s); }; /* 请在这里填写答案 */ ostream& operator <<(ostream &out, Student &s) { out << "ID:" << s.id << " 姓名:" << s.name << " 专业:" << s.major << " 成绩:" << s.score; return out; } int main() { Student a("ypc202101", "胡某某", "电子信息专业", 95); cout << a << endl; return 0; } 输入样例: 无 输出样例: ID:ypc202101 姓名:胡某某 专业:电子信息专业 成绩:95
时间: 2024-01-27 13:06:21 浏览: 39
下面是题目的 C++ 代码实现:
```c++
#include <iostream>
#include <string>
using namespace std;
class Person {
protected:
string id;
string name;
public:
Person(string id, string name) {
this->id = id;
this->name = name;
}
};
class Student : public Person {
private:
string major;
int score;
public:
Student(string id, string name, string major, int score)
: Person(id, name) {
this->major = major;
this->score = score;
}
friend ostream& operator<<(ostream& out, Student& s);
};
ostream& operator<<(ostream& out, Student& s) {
out << "ID:" << s.id << " 姓名:" << s.name
<< " 专业:" << s.major << " 成绩:" << s.score;
return out;
}
int main() {
Student a("ypc202101", "胡某某", "电子信息专业", 95);
cout << a << endl;
return 0;
}
```
在 `Student` 类的构造函数中,需要调用 `Person` 类的构造函数来初始化 `id` 和 `name` 成员变量。在输出 `Student` 对象时,需要重载 `<<` 运算符来自定义输出格式。在 `main` 函数中,创建一个 `Student` 对象并输出。
相关推荐
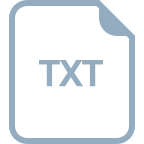
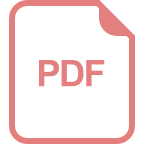
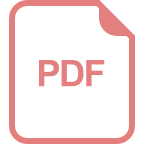









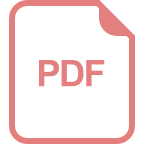
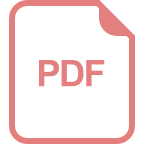
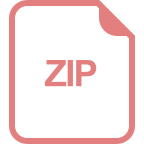
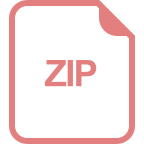
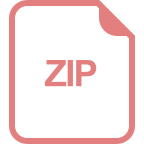