给我写个顺序表的学生管理系统
时间: 2023-05-24 14:06:39 浏览: 45
以下是一个基本的顺序表学生管理系统的实现:
```
#include<iostream>
using namespace std;
//定义学生结构体
struct student{
int no; //学号
string name; //姓名
int age; //年龄
double score; //成绩
};
//定义学生管理类
class studentList{
private:
student *stuList; //学生数组
int maxSize; //最大容量
int length; //当前长度
public:
studentList(int size){ //初始化构造函数
maxSize = size;
stuList = new student[maxSize];
length = 0;
}
~studentList(){ //析构函数
delete []stuList;
}
void clearList(){ //清空表
length = 0;
}
bool listEmpty(){ //判空
return length == 0;
}
int listLength(){ //表长度
return length;
}
bool getElem(int i, student &s){ //获取指定位置元素
if(i < 1 || i > length){
return false;
}
s = stuList[i-1];
return true;
}
int locateElem(student s){ //查找元素位置
for(int i=0; i<length; i++){
if(stuList[i].no == s.no){
return i+1;
}
}
return 0;
}
bool listInsert(int i, student s){ //插入元素
if(i < 1 || i > length+1 || length == maxSize){
return false;
}
for(int j=length-1; j>=i-1; j--){
stuList[j+1] = stuList[j];
}
stuList[i-1] = s;
length++;
return true;
}
bool listDelete(int i){ //删除元素
if(i < 1 || i > length){
return false;
}
for(int j=i; j<length; j++){
stuList[j-1] = stuList[j];
}
length--;
return true;
}
void displayList(){ //遍历表
student s;
for(int i=0; i<length; i++){
s = stuList[i];
cout << "No." << s.no << "\tName:" << s.name << "\tAge:" << s.age << "\tScore:" << s.score << endl;
}
cout << endl;
}
};
//主函数
int main(){
studentList sl(100); //定义学生管理对象
student s; //定义学生对象
s.no = 101;
s.name = "Tom";
s.age = 18;
s.score = 91.5;
sl.listInsert(1, s); //插入第一个学生
s.no = 102;
s.name = "Lucy";
s.age = 19;
s.score = 89.5;
sl.listInsert(2, s); //插入第二个学生
s.no = 103;
s.name = "Mike";
s.age = 20;
s.score = 87.5;
sl.listInsert(3, s); //插入第三个学生
cout << "The list is:" << endl;
sl.displayList(); //遍历学生表
s.no = 102;
int i = sl.locateElem(s);
if(i == 0){
cout << "Can not find student 102" << endl;
}else{
cout << "Student 102 was found at " << i << endl;
}
cout << "Delete the second student:" << endl;
sl.listDelete(2); //删除第二个学生
cout << "The list is:" << endl;
sl.displayList(); //遍历学生表
sl.clearList(); //清空学生表
cout << "After clear, the list is:";
cout << sl.listEmpty() << endl; //判空
return 0;
}
```
在上面的实现中,我们首先定义了一个学生结构体,其中包含学号、姓名、年龄和成绩四个属性。接着定义了一个学生管理类studentList,其中包含了许多常用的操作函数,包括清空表、判空、表长度、获取指定位置元素、查找元素位置、插入元素、删除元素等等。
在主函数中,我们首先创建了一个学生管理对象sl,并依次插入了三个学生记录。然后我们需要遍历学生表,可以调用displayList()函数来实现。接着,我们对学生表进行了查询操作,调用了locateElem()函数查找学号为102的学生,并输出了其位置信息。然后我们又删除了表中的第二个学生记录,并输出了删除后的结果。最后,我们清空了整个学生表,并输出了是否为空的结果。
相关推荐
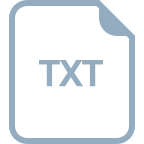
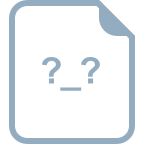














