在vue中使用echarts实现饼状图动态数据
时间: 2023-11-07 18:05:15 浏览: 43
实现饼状图动态数据的过程分为两步:
1. 初始化echarts实例,并设置饼状图的基本配置。
2. 在Vue组件中通过异步请求数据,将数据传入到echarts实例中,动态更新饼状图的数据。
下面是一个简单的示例代码:
```html
<template>
<div id="pieChart" style="width: 100%; height: 400px;"></div>
</template>
<script>
import echarts from 'echarts';
export default {
data() {
return {
pieChart: null,
pieData: [
{ name: 'A', value: 0 },
{ name: 'B', value: 0 },
{ name: 'C', value: 0 },
],
};
},
mounted() {
this.initPieChart();
},
methods: {
initPieChart() {
// 初始化echarts实例
this.pieChart = echarts.init(document.getElementById('pieChart'));
// 设置饼状图基本配置
this.pieChart.setOption({
title: { text: '饼状图示例' },
tooltip: { trigger: 'item' },
legend: { data: ['A', 'B', 'C'] },
series: [
{
name: '数据',
type: 'pie',
radius: '55%',
center: ['50%', '60%'],
data: this.pieData,
itemStyle: {
emphasis: {
shadowBlur: 10,
shadowOffsetX: 0,
shadowColor: 'rgba(0, 0, 0, 0.5)',
},
},
},
],
});
},
async fetchData() {
// 异步请求数据
const response = await fetch('http://example.com/data');
const data = await response.json();
// 更新饼状图数据
this.pieData = [
{ name: 'A', value: data.a },
{ name: 'B', value: data.b },
{ name: 'C', value: data.c },
];
this.pieChart.setOption({
series: [
{
data: this.pieData,
},
],
});
},
},
};
</script>
```
在mounted方法中初始化echarts实例,并在initPieChart方法中设置饼状图的基本配置。然后在fetchData方法中异步请求数据,将数据传入到echarts实例中,动态更新饼状图的数据。最后,在模板中渲染一个div元素作为echarts的容器。
相关推荐
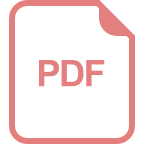
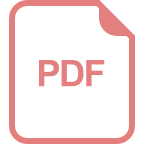
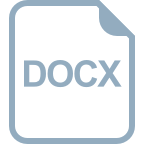
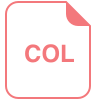
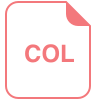
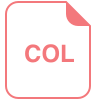
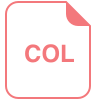
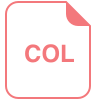









