如何用python打开一个二进制文件,它使用多种编码格式混合而成,如何打印出里面GB2312,GB18030,GBK,BIG5,unicode,utf-8,utf-16 be,utf-16le格式的中文汉字
时间: 2023-09-10 09:05:03 浏览: 134
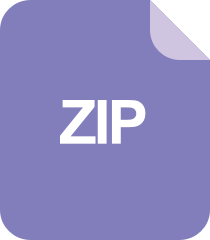
字符编码UTF8转GB2312+可批量转换+需安装Python
### 回答1:
可以使用 Python 的 `codecs` 库来打开二进制文件并读取它的内容。
下面是一个示例代码:
```
import codecs
# 打开文件,以二进制模式读取
with codecs.open('binary_file.bin', 'rb') as f:
# 读取文件内容
data = f.read()
# 尝试使用多种编码格式解码文件内容
try:
# GB2312
text = data.decode('gb2312')
print(text)
except UnicodeDecodeError:
pass
try:
# GB18030
text = data.decode('gb18030')
print(text)
except UnicodeDecodeError:
pass
try:
# GBK
text = data.decode('gbk')
print(text)
except UnicodeDecodeError:
pass
try:
# BIG5
text = data.decode('big5')
print(text)
except UnicodeDecodeError:
pass
try:
# Unicode
text = data.decode('unicode')
print(text)
except UnicodeDecodeError:
pass
try:
# UTF-8
text = data.decode('utf-8')
print(text)
except UnicodeDecodeError:
pass
try:
# UTF-16 BE
text = data.decode('utf-16-be')
print(text)
except UnicodeDecodeError:
pass
try:
# UTF-16 LE
text = data.decode('utf-16-le')
print(text)
except UnicodeDecodeError:
pass
```
在这段代码中,我们首先使用 `codecs.open` 函数打开文件,并以二进制模式读取。然后,我们使用 `data.decode` 函数尝试使用多种编码格式对文件内容进行解码。如果某一种编码格式不能正确解码,就会抛出 `UnicodeDecodeError` 异常,我们可以使用 `except` 语句捕获这个异常,并忽略它。
在这段代
### 回答2:
在Python中,可以使用`open()`函数来打开一个二进制文件,并使用不同的编码格式来打印出其中的中文汉字。
首先,使用`open()`函数打开二进制文件,并设置`'rb'`参数以二进制读取模式打开文件。
```python
with open('filename', 'rb') as f:
content = f.read()
```
接下来,可以使用`decode()`函数将二进制数据解码成字符串,并指定相应的编码格式。
```python
content_gb2312 = content.decode('gb2312')
content_gb18030 = content.decode('gb18030')
content_gbk = content.decode('gbk')
content_big5 = content.decode('big5')
content_unicode = content.decode('unicode_escape')
content_utf8 = content.decode('utf-8')
content_utf16be = content.decode('utf-16be')
content_utf16le = content.decode('utf-16le')
```
最后,可以使用`print()`函数打印出解码后的字符串。
```python
print(content_gb2312)
print(content_gb18030)
print(content_gbk)
print(content_big5)
print(content_unicode)
print(content_utf8)
print(content_utf16be)
print(content_utf16le)
```
这样就可以打印出二进制文件中各种编码格式的中文汉字了。当然,需要确保打开文件时使用正确的编码格式。
### 回答3:
要打开一个二进制文件并打印出其中不同编码格式的中文汉字,可以按照以下步骤进行:
1. 使用Python的`open()`函数打开二进制文件,指定文件路径和读取模式。例如,`file = open('file.bin', 'rb')`。
2. 读取文件内容,可以使用`file.read()`方法将文件内容读取为字节串。
3. 将字节串转换为不同的编码格式的字符串进行打印。使用Python的`decode()`方法可以将字节串解码为指定编码格式的字符串。例如,使用`data.decode('gb2312')`将字节串解码为GB2312编码格式的字符串。
4. 重复步骤3,使用不同的编码格式将字节串解码为字符串,分别打印出中文汉字。
下面是一个示例代码:
```python
file = open('file.bin', 'rb')
data = file.read()
encodings = ['gb2312', 'gb18030', 'gbk', 'big5', 'unicode', 'utf-8', 'utf-16be', 'utf-16le']
for encoding in encodings:
try:
decoded_data = data.decode(encoding)
print(f'{encoding}: {decoded_data}')
except UnicodeDecodeError:
print(f'Error decoding with {encoding} encoding')
file.close()
```
在上述代码中,使用一个包含不同编码格式的列表`encodings`,依次将字节串解码为字符串并打印出来。如果某个编码格式无法正确解码,会捕获并处理`UnicodeDecodeError`异常。
请注意,要根据实际情况修改文件路径和文件名,确保打开的是正确的二进制文件。
阅读全文
相关推荐
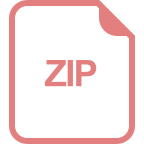
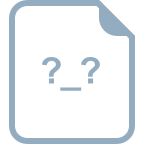

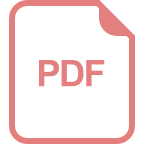
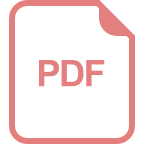
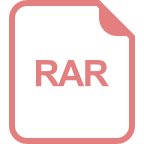
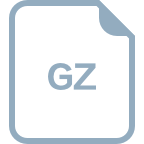
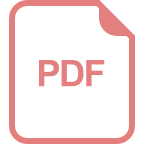
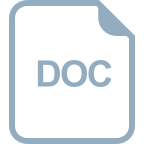
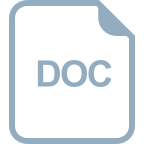
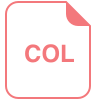
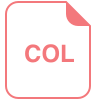
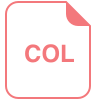
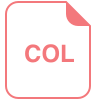
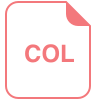
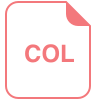
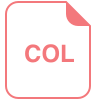