Python 对FTP文件夹内的txt文件进行合并处理
时间: 2024-12-23 13:13:25 浏览: 6
在Python中,你可以使用`ftplib`库来连接到FTP服务器并下载TXT文件,然后使用内置的字符串操作或`csv`、`pandas`等库来进行合并处理。以下是一个简单的步骤:
1. **导入必要的库**:
```python
import ftplib
import os
# 可选:用于文本处理
import glob
# 或者用于CSV合并(如果TXT文件包含逗号分隔的数据)
import pandas as pd
```
2. **连接到FTP服务器**:
```python
ftp = ftplib.FTP('ftp.example.com', 'username', 'password') # 替换为实际的服务器地址和凭证
ftp.login()
```
3. **列出文件夹中的TXT文件**:
```python
def list_files(dir_path):
return [f for f in ftp.nlst(dir_path) if f.endswith('.txt')]
txt_files = list_files('/')
```
4. **下载TXT文件并临时保存**:
```python
temp_dir = './temp'
if not os.path.exists(temp_dir):
os.makedirs(temp_dir)
for file in txt_files:
with open(os.path.join(temp_dir, file), 'wb') as local_file:
ftp.retrbinary(f'RETR {file}', local_file.write)
```
5. **合并文件**:
- 如果是简单的文本合并,可以使用`open`函数逐行读取每个文件,然后追加到一个大文件中:
```python
output_file = 'merged.txt'
with open(output_file, 'w') as outfile:
for filename in temp_dir + '/':
with open(filename, 'r') as infile:
outfile.write(infile.read())
```
- 如果TXT文件是CSV格式,使用`pd.concat`:
```python
data_frames = [pd.read_csv(os.path.join(temp_dir, f)) for f in txt_files]
merged_df = pd.concat(data_frames, ignore_index=True)
merged_df.to_csv('merged.csv', index=False)
```
6. **关闭连接**:
```python
ftp.quit()
```
记得替换上述代码中的URL、用户名、密码以及路径,根据你的实际情况调整。
阅读全文
相关推荐
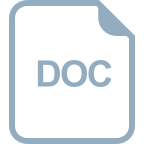
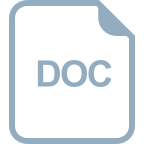
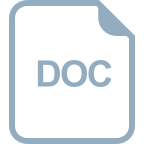
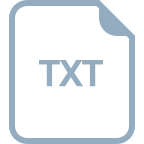
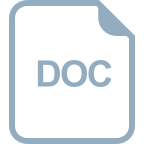
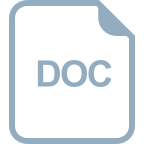
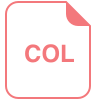
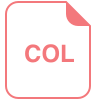
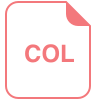
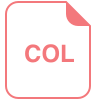
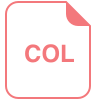
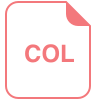
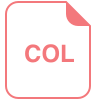
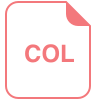
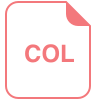
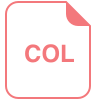
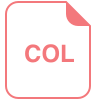
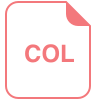
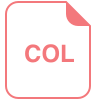
