写出无约束变尺度法c语言程序,并举f(x)=x1^2+25*x2^2的例子
时间: 2024-12-16 15:15:47 浏览: 11
在C语言中,无约束变尺度法的具体实现可能会比较复杂,因为它涉及迭代和矩阵运算,这通常在库函数中处理。然而,我们可以创建一个简单的伪代码来展示基本思路。这里我们将使用一个非常基础的版本,仅用于演示,实际应用中可能需要结合数值优化库或者使用矩阵库。
```c
#include <stdio.h>
#include <math.h>
// 定义目标函数及梯度
double f(double *x) {
double x1 = x[0], x2 = x[1];
return x1 * x1 + 25 * x2 * x2;
}
void gradient(double *x, double *grad) {
grad[0] = 2 * x[0]; // df/dx1
grad[1] = 50 * x[1]; // df/dx2
}
// 变尺度函数
void variable_scaling(double *x, double *scale_factors) {
double norm = sqrt(scale_factors[0] * scale_factors[0] + scale_factors[1] * scale_factors[1]);
if (norm > 0) {
scale_factors[0] /= norm;
scale_factors[1] /= norm;
}
}
int main() {
double x[2] = {0.5, 0.5}; // 初始点
double grad[2]; // 梯度
double step_size = 0.01; // 学习率
double scale_factors[2] = {1, 1}; // 初始尺度
while (/* 某些收敛条件未满足 */) {
gradient(x, grad); // 计算梯度
variable_scaling(x, scale_factors); // 应用变尺度
for (int i = 0; i < 2; ++i) {
x[i] -= scale_factors[i] * step_size * grad[i]; // 更新x
}
printf("Current point: (%lf, %lf), Function value: %.6lf\n", x[0], x[1], f(x));
}
return 0;
}
```
这个例子只是一个简化的模型,实际使用时需要考虑更多细节,比如如何设置收敛条件,如何处理边界值等。同时,为了性能和精度,一般建议使用专门的数值优化库,如GNU Scientific Library (GSL) 或 Armadillo。
阅读全文
相关推荐






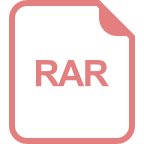
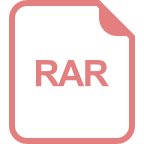




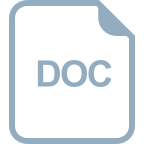

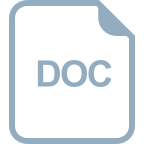


