写出实现双向链表的建表、销毁表、清空表、判断空、求表长度、按值查找等基本操作的C语言程序代码
时间: 2023-09-28 08:11:15 浏览: 79
双向链表结构体定义:
```c
typedef struct DuLNode {
ElemType data; // 数据域
struct DuLNode *prior; // 指向前驱结点
struct DuLNode *next; // 指向后继结点
} DuLNode, *DuLinkedList;
```
建表操作:
```c
void CreateList(DuLinkedList *L, int n) {
*L = (DuLinkedList) malloc(sizeof(DuLNode));
(*L)->prior = NULL;
(*L)->next = NULL;
DuLinkedList p = *L;
for (int i = 0; i < n; i++) {
DuLinkedList q = (DuLinkedList) malloc(sizeof(DuLNode));
scanf("%d", &q->data);
q->prior = p;
q->next = p->next;
p->next = q;
p = q;
}
}
```
销毁表操作:
```c
void DestroyList(DuLinkedList *L) {
DuLinkedList p = (*L)->next;
while (p != NULL) {
DuLinkedList q = p->next;
free(p);
p = q;
}
(*L)->next = NULL;
}
```
清空表操作:
```c
void ClearList(DuLinkedList L) {
DuLinkedList p = L->next;
while (p != NULL) {
DuLinkedList q = p->next;
free(p);
p = q;
}
L->next = NULL;
}
```
判断空操作:
```c
bool ListIsEmpty(DuLinkedList L) {
return L->next == NULL;
}
```
求表长度操作:
```c
int ListLength(DuLinkedList L) {
int len = 0;
DuLinkedList p = L->next;
while (p != NULL) {
len++;
p = p->next;
}
return len;
}
```
按值查找操作:
```c
DuLinkedList GetElem(DuLinkedList L, ElemType e) {
DuLinkedList p = L->next;
while (p != NULL && p->data != e) {
p = p->next;
}
return p;
}
```
阅读全文
相关推荐

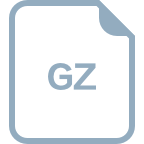

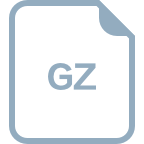








