二进制文件的加密解密 c
时间: 2024-01-02 11:03:11 浏览: 25
二进制文件加密解密可以使用对称加密算法,比如AES。下面是一个使用C语言实现的例子:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/aes.h>
#define AES_KEY_SIZE 128
#define BLOCK_SIZE 16
void encrypt_file(char* input_file, char* output_file, unsigned char* key, unsigned char* iv) {
AES_KEY aes_key;
if (AES_set_encrypt_key(key, AES_KEY_SIZE, &aes_key) < 0) {
printf("Failed to set encrypt key\n");
exit(1);
}
FILE* in_fp = fopen(input_file, "rb");
FILE* out_fp = fopen(output_file, "wb");
unsigned char in_buf[BLOCK_SIZE];
unsigned char out_buf[BLOCK_SIZE];
int bytes_read, bytes_written;
while ((bytes_read = fread(in_buf, 1, BLOCK_SIZE, in_fp)) > 0) {
AES_cbc_encrypt(in_buf, out_buf, bytes_read, &aes_key, iv, AES_ENCRYPT);
bytes_written = fwrite(out_buf, 1, bytes_read, out_fp);
if (bytes_written != bytes_read) {
printf("Failed to write encrypted data\n");
exit(1);
}
}
fclose(in_fp);
fclose(out_fp);
}
void decrypt_file(char* input_file, char* output_file, unsigned char* key, unsigned char* iv) {
AES_KEY aes_key;
if (AES_set_decrypt_key(key, AES_KEY_SIZE, &aes_key) < 0) {
printf("Failed to set decrypt key\n");
exit(1);
}
FILE* in_fp = fopen(input_file, "rb");
FILE* out_fp = fopen(output_file, "wb");
unsigned char in_buf[BLOCK_SIZE];
unsigned char out_buf[BLOCK_SIZE];
int bytes_read, bytes_written;
while ((bytes_read = fread(in_buf, 1, BLOCK_SIZE, in_fp)) > 0) {
AES_cbc_encrypt(in_buf, out_buf, bytes_read, &aes_key, iv, AES_DECRYPT);
bytes_written = fwrite(out_buf, 1, bytes_read, out_fp);
if (bytes_written != bytes_read) {
printf("Failed to write decrypted data\n");
exit(1);
}
}
fclose(in_fp);
fclose(out_fp);
}
int main() {
unsigned char key[AES_KEY_SIZE / 8] = {0x00, 0x01, 0x02, 0x03, 0x04, 0x05, 0x06, 0x07, 0x08, 0x09, 0x0a, 0x0b, 0x0c, 0x0d, 0x0e, 0x0f};
unsigned char iv[BLOCK_SIZE] = {0x10, 0x11, 0x12, 0x13, 0x14, 0x15, 0x16, 0x17, 0x18, 0x19, 0x1a, 0x1b, 0x1c, 0x1d, 0x1e, 0x1f};
char* input_file = "input.bin";
char* encrypted_file = "encrypted.bin";
char* decrypted_file = "decrypted.bin";
// 加密
encrypt_file(input_file, encrypted_file, key, iv);
// 解密
decrypt_file(encrypted_file, decrypted_file, key, iv);
return 0;
}
```
该例子中使用了AES对称加密算法,加密模式为CBC。其中key和iv可以自行生成,input_file为要加密的二进制文件,encrypted_file为加密后的文件,decrypted_file为解密后的文件。在实际使用中,可以将key和iv保存在安全的地方,以供解密时使用。
相关推荐
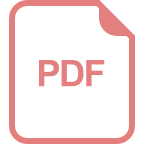
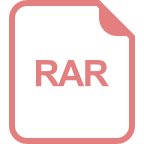














