y_test = y_test.reset_index(drop = True) y_predict2 = model_RF1.predict(x_test) plt.figure(figsize = (12,8)) plt.plot(y_predict2,color = 'b',label = 'predict',markersize=8) plt.plot(y_test,color = 'r',label = 'true',markersize=8) plt.xlabel('Test Sample',fontsize=30) plt.ylabel('y1',fontsize=30) plt.title('随机森林',fontsize=30) #坐标轴字体大小 plt.xticks(fontsize=25) plt.yticks(fontsize=25) plt.legend(fontsize=25,loc='upper right') # plt.savefig('GradientBoosting.png',dpi=300,bbox_inches = 'tight')
时间: 2024-01-02 16:05:01 浏览: 37
这段代码的作用是对训练好的随机森林模型进行测试,并将测试结果和真实值进行可视化比较。首先,使用`reset_index()`函数将`y_test`的索引重置为默认值,即0到n-1。接着,使用训练好的`model_RF1`模型对测试集`x_test`进行预测,将预测结果存储在`y_predict2`中。然后,使用`plt.plot()`函数将预测结果和真实值分别用蓝色和红色的线绘制在图表上。接下来,使用`plt.xlabel()`和`plt.ylabel()`函数分别设置x轴和y轴的标签,使用`plt.title()`函数设置图表的标题。然后,使用`plt.xticks()`和`plt.yticks()`函数设置x轴和y轴刻度标签的字体大小。最后,使用`plt.legend()`函数添加图例,并设置字体大小和位置。如果需要保存图表,可以使用`plt.savefig()`函数。
相关问题
y_pred = model.predict(X_test)
这段代码是在测试数据集(X_test)上使用训练好的决策树分类器模型(model)进行预测。具体来说,使用predict()方法对测试数据集进行预测,并将预测结果存储在变量y_pred中。y_pred是一个数组,其中包含测试数据集中每个样本的预测标签。通过比较y_pred和测试数据集的真实标签,可以评估模型的准确性和性能。
改进y_predict = lr.predict(x_test)
您可以尝试使用交叉验证(cross-validation)来改进y_predict = lr.predict(x_test)。交叉验证可以帮助您更准确地评估模型的性能,从而避免过拟合的问题。您可以使用sklearn库中的cross_val_score()函数进行交叉验证,具体操作可以参考相关文档。
相关推荐
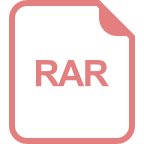














