python查找目录下多个后缀文件,需要排除掉一些目录。
时间: 2024-05-14 21:12:59 浏览: 107
可以使用os.walk()函数遍历目录下的所有子目录和文件,使用endswith()方法匹配需要查找的后缀名,使用if语句排除不需要查找的目录。
示例代码如下:
```python
import os
# 需要查找的后缀名
extensions = ['.txt', '.csv', '.log']
# 不需要查找的目录
exclude_dirs = ['tmp', 'cache']
# 遍历目录
for root, dirs, files in os.walk('.'):
# 排除不需要查找的目录
dirs[:] = [d for d in dirs if d not in exclude_dirs]
for file in files:
# 匹配需要查找的后缀名
if file.endswith(tuple(extensions)):
# 处理找到的文件
print(os.path.join(root, file))
```
在上面的代码中,os.walk()函数返回一个三元组,包含当前遍历的目录路径、该目录下的子目录列表和该目录下的文件列表。使用os.path.join()函数拼接目录路径和文件名,以获取文件的完整路径。
相关问题
python查找目录下文件,需要排除掉一些目录。找到多个文件后缀
为.py的文件。
可以使用os模块和fnmatch模块来实现此功能。
代码示例:
```python
import os
import fnmatch
# 需要排除的目录列表
exclude_dirs = ['dir1', 'dir2']
# 查找的文件后缀
file_extension = '.py'
# 查找目录
search_dir = '/path/to/search/dir'
# 遍历目录及其子目录
for root, dirs, files in os.walk(search_dir):
# 排除需要排除的目录
dirs[:] = [d for d in dirs if d not in exclude_dirs]
# 查找符合条件的文件
for filename in fnmatch.filter(files, f'*{file_extension}'):
# 打印文件路径
print(os.path.join(root, filename))
```
在以上代码中,首先定义了需要排除的目录列表和要查找的文件后缀。然后使用os.walk()函数遍历目录及其子目录,在遍历过程中排除需要排除的目录,并使用fnmatch.filter()函数查找符合条件的文件,最后打印文件路径。
阅读全文
相关推荐
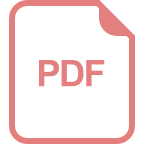
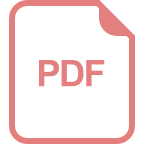
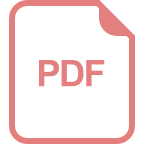
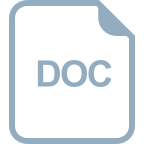
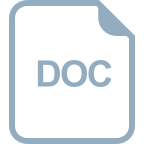
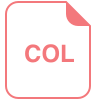
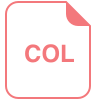
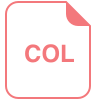
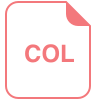
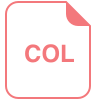
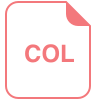
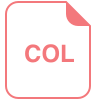
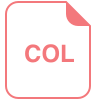
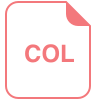
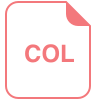
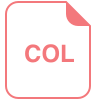
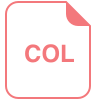
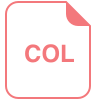