java对文件中的若干个字符串进行基数排序,并将排序后的字符串更新到文件中。
时间: 2024-05-01 07:20:59 浏览: 53
基数排序是一种非常高效的排序算法,可以用于对字符串进行排序。下面是一个 Java 实现示例:
```java
import java.io.*;
import java.util.*;
public class RadixSort {
public static void main(String[] args) throws IOException {
File file = new File("input.txt");
BufferedReader reader = new BufferedReader(new FileReader(file));
// 读取文件中的所有字符串
List<String> strings = new ArrayList<>();
String line;
while ((line = reader.readLine()) != null) {
strings.add(line);
}
reader.close();
// 计算字符串的最大长度
int maxLength = 0;
for (String s : strings) {
maxLength = Math.max(maxLength, s.length());
}
// 从低位到高位依次对字符串进行排序
for (int i = 0; i < maxLength; i++) {
List<List<String>> buckets = new ArrayList<>(10);
for (int j = 0; j < 10; j++) {
buckets.add(new ArrayList<>());
}
for (String s : strings) {
int index = i < s.length() ? Character.digit(s.charAt(s.length() - i - 1), 10) : 0;
buckets.get(index).add(s);
}
strings.clear();
for (List<String> bucket : buckets) {
strings.addAll(bucket);
}
}
// 将排序后的字符串写回文件
BufferedWriter writer = new BufferedWriter(new FileWriter(file));
for (String s : strings) {
writer.write(s);
writer.newLine();
}
writer.close();
}
}
```
这个程序首先读取文件中的所有字符串,然后计算字符串的最大长度,接着从低位到高位依次对字符串进行排序。在每一轮排序中,我们将字符串按照当前位的数值分配到 10 个桶中,然后按照桶的顺序重新组合成新的字符串列表。最后,将排序后的字符串写回文件。
相关推荐
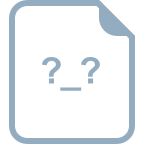














