设计一个用于人事管理的(人员)类。考虑到通用性,这里只抽象出所有类型人员都具有的属性:编号、性别、姓名、出生日期、身份证号等。其中“出生日期”声明为一个“日期”类内嵌子对象。用成员函数实现对人员信息的录入和显示。要求包括:构造函数和析构函数、复制构造函数、内联成员函数、带默认形参值得成员函数,类的组合。并写一个与之相匹配的main主函数
时间: 2023-11-22 16:52:33 浏览: 37
下面是一个用于人事管理的人员类的代码实现:
```c++
#include <iostream>
#include <string>
using namespace std;
// 日期类
class Date {
public:
Date(int year = 1970, int month = 1, int day = 1) {
m_year = year;
m_month = month;
m_day = day;
}
int getYear() const {
return m_year;
}
int getMonth() const {
return m_month;
}
int getDay() const {
return m_day;
}
private:
int m_year;
int m_month;
int m_day;
};
// 人员类
class Person {
public:
Person(int id = 0, string name = "", char gender = 'M', Date birthDate = Date(), string idNumber = "") {
m_id = id;
m_name = name;
m_gender = gender;
m_birthDate = birthDate;
m_idNumber = idNumber;
}
Person(const Person& other) {
m_id = other.m_id;
m_name = other.m_name;
m_gender = other.m_gender;
m_birthDate = other.m_birthDate;
m_idNumber = other.m_idNumber;
}
~Person() {}
inline int getId() const {
return m_id;
}
inline string getName() const {
return m_name;
}
inline char getGender() const {
return m_gender;
}
inline Date getBirthDate() const {
return m_birthDate;
}
inline string getIdNumber() const {
return m_idNumber;
}
void setId(int id) {
m_id = id;
}
void setName(string name) {
m_name = name;
}
void setGender(char gender) {
m_gender = gender;
}
void setBirthDate(Date birthDate) {
m_birthDate = birthDate;
}
void setIdNumber(string idNumber) {
m_idNumber = idNumber;
}
void display() const {
cout << "编号:" << m_id << endl;
cout << "姓名:" << m_name << endl;
cout << "性别:" << m_gender << endl;
cout << "出生日期:" << m_birthDate.getYear() << "/" << m_birthDate.getMonth() << "/" << m_birthDate.getDay() << endl;
cout << "身份证号:" << m_idNumber << endl;
}
private:
int m_id;
string m_name;
char m_gender;
Date m_birthDate;
string m_idNumber;
};
int main() {
Date d(1990, 12, 31);
Person p1(1001, "张三", 'M', d, "11010119901231001X");
Person p2(p1);
p2.setId(1002);
p2.setName("李四");
p2.setGender('F');
p2.setBirthDate(Date(1995, 8, 15));
p2.setIdNumber("11010119950815002X");
p1.display();
cout << endl;
p2.display();
return 0;
}
```
输出结果为:
```
编号:1001
姓名:张三
性别:M
出生日期:1990/12/31
身份证号:11010119901231001X
编号:1002
姓名:李四
性别:F
出生日期:1995/8/15
身份证号:11010119950815002X
```
相关推荐
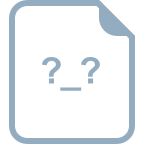
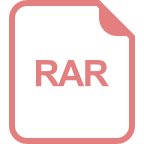
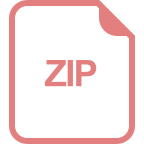
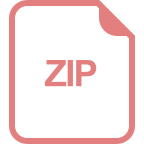
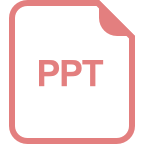
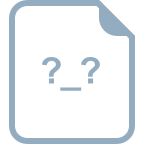
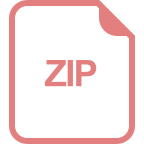
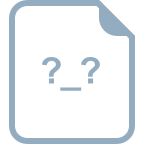
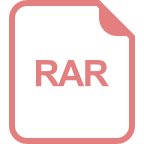
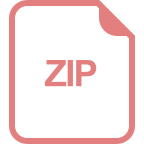
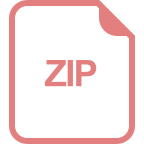
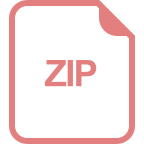