1.读取CSV文件Output 2.把标题行分为4类,第一类是标题为ABC,DFG,TBC,DHHG,lop,kki的6列,第二类是CAT开头的几列,第三类是DOG开头的几列,第四类是Fish和food开头的几列 3.在一张画布上分成4份,把4类标题画成4个曲线图,标注每条线的标题
时间: 2024-03-07 07:46:34 浏览: 89
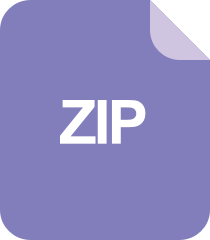
DFG.zip_2D_2D explicit matlab_explicit_heat_heat transfer
好的,您的问题是如何实现读取CSV文件,并将标题行分为4类,然后将它们绘制成4个曲线图并标注标题。我可以为您提供一些思路和Python代码示例。
首先,您可以使用Python内置的csv模块来读取CSV文件。下面是一个简单的示例,可以读取名为“file.csv”的文件并打印其中的数据:
```python
import csv
with open('file.csv', newline='') as csvfile:
reader = csv.reader(csvfile, delimiter=',', quotechar='"')
for row in reader:
print(row)
```
接下来,您需要将标题行分为4类。您可以使用Python的字符串方法来检查标题是否以特定的字符串开头。下面是一个简单的示例,可以将标题分为4类并将它们打印出来:
```python
category1 = ['ABC', 'DFG', 'TBC', 'DHHG', 'lop', 'kki']
category2 = ['CAT']
category3 = ['DOG']
category4 = ['Fish', 'food']
with open('file.csv', newline='') as csvfile:
reader = csv.reader(csvfile, delimiter=',', quotechar='"')
headers = next(reader)
for header in headers:
if header in category1:
print(header, 'belongs to category 1')
elif header.startswith(tuple(category2)):
print(header, 'belongs to category 2')
elif header.startswith(tuple(category3)):
print(header, 'belongs to category 3')
elif header.startswith(tuple(category4)):
print(header, 'belongs to category 4')
```
最后,您可以使用Python的Matplotlib库来绘制4个曲线图。下面是一个简单的示例,可以将每个类别的数据绘制成一个曲线图:
```python
import matplotlib.pyplot as plt
category1_data = []
category2_data = []
category3_data = []
category4_data = []
with open('file.csv', newline='') as csvfile:
reader = csv.reader(csvfile, delimiter=',', quotechar='"')
headers = next(reader)
for row in reader:
category1_data.append(float(row[headers.index('ABC')]))
category2_data.append(float(row[headers.index('CAT1')]) + float(row[headers.index('CAT2')]))
category3_data.append(float(row[headers.index('DOG1')]) + float(row[headers.index('DOG2')]))
category4_data.append(float(row[headers.index('Fish1')]) + float(row[headers.index('Fish2')]) + float(row[headers.index('food')]))
plt.subplot(2, 2, 1)
plt.plot(category1_data)
plt.title('Category 1')
plt.subplot(2, 2, 2)
plt.plot(category2_data)
plt.title('Category 2')
plt.subplot(2, 2, 3)
plt.plot(category3_data)
plt.title('Category 3')
plt.subplot(2, 2, 4)
plt.plot(category4_data)
plt.title('Category 4')
plt.show()
```
这个示例假设您的CSV文件中有ABC、CAT1、CAT2、DOG1、DOG2、Fish1、Fish2和food这些列,并且它们都包含数字数据。您可以根据您的实际情况进行调整。
希望这些示例能够帮助您解决问题!
阅读全文
相关推荐
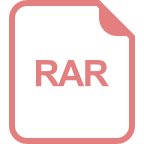
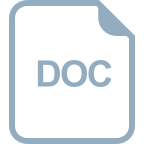


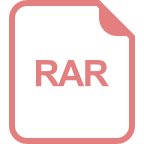
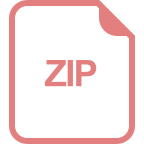
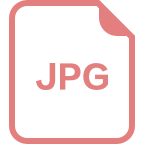
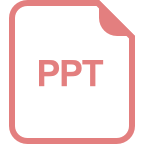
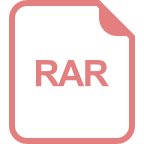
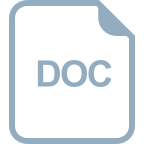
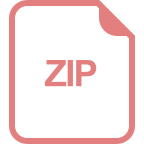
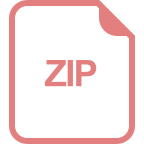
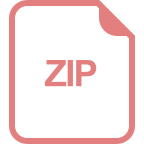
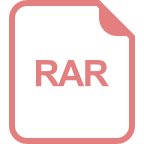
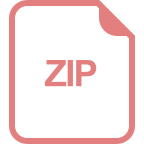