c++编写程序用于水果销售算账。已知苹果每斤5.5元,鸭梨每斤3.8元,香蕉每斤4.5元,橘子每斤4元。要求输入各类水果的数量,输出应付的钱数,再根据顾客所付的钱数,输出应找的钱数。
时间: 2024-12-22 08:29:44 浏览: 21
在C++中,你可以创建一个简单的控制台应用来解决这个问题。首先,我们可以定义一个结构体来存储每种水果的信息,然后定义函数来计算总价格、找零等。以下是基本的代码框架:
```cpp
#include <iostream>
using namespace std;
// 定义水果信息结构
struct Fruit {
string name;
double price;
};
// 计算总价的函数
double calculateTotalPrice(Fruit* fruits, int count) {
double total = 0;
for (int i = 0; i < count; i++) {
total += fruits[i].price;
}
return total;
}
// 计算找零的函数
double calculateChange(double payment, double totalPrice) {
return payment - totalPrice;
}
int main() {
// 定义水果及价格
Fruit fruitList[] = {{"苹果", 5.5}, {"鸭梨", 3.8}, {"香蕉", 4.5}, {"橘子", 4}};
const int numFruits = sizeof(fruitList) / sizeof(fruitList[0]);
// 输入用户购买的数量
int counts[numFruits];
for (int i = 0; i < numFruits; i++) {
cout << "请输入第" << i+1 << "种水果的数量: ";
cin >> counts[i];
}
// 计算总价和找零
double payment;
cout << "请输入付款金额: ";
cin >> payment;
double totalPrice = calculateTotalPrice(fruitList, numFruits);
double change = calculateChange(payment, totalPrice);
// 输出结果
cout << "总价: " << totalPrice << endl;
cout << "找零: " << change << endl;
return 0;
}
```
阅读全文
相关推荐







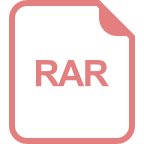
