C语言 字符串去重排序
时间: 2023-09-02 21:12:04 浏览: 227
可以使用以下步骤来实现字符串去重排序:
1. 定义一个字符数组,用于存储去重后的字符。
2. 遍历原始字符串中的每个字符,判断该字符是否已经存在于字符数组中。
3. 如果不存在,则将该字符添加到字符数组中。
4. 对字符数组进行排序。
5. 将字符数组转换为字符串输出。
以下是一个示例代码:
```c
#include <stdio.h>
#include <string.h>
void remove_duplicates(char *str) {
int len = strlen(str);
char res[len];
int idx = 0;
for (int i = 0; i < len; i++) {
int j;
for (j = 0; j < idx; j++) {
if (res[j] == str[i]) {
break;
}
}
if (j == idx) {
res[idx++] = str[i];
}
}
res[idx] = '\0';
// 排序
qsort(res, idx, sizeof(char), cmp);
printf("%s\n", res);
}
int cmp(const void *a, const void *b) {
return *(char *)a - *(char *)b;
}
int main() {
char str[] = "abbcdeeff";
remove_duplicates(str);
return 0;
}
```
这个程序的输出结果是:abcdef。
阅读全文
相关推荐
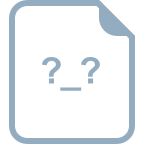
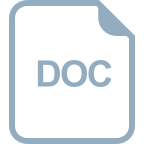
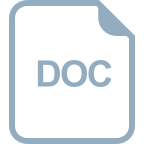
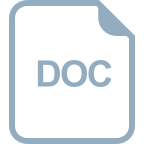





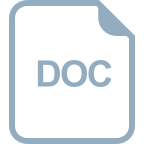
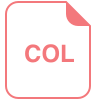
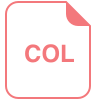




