static float prob[BATCH_SIZE * OUTPUT_SIZE]
时间: 2023-12-28 11:04:39 浏览: 109
这是一个静态的 float 数组,大小为 BATCH_SIZE * OUTPUT_SIZE。根据命名和常见约定,它很可能是用于存储模型对输入数据的预测结果的概率值。BATCH_SIZE 表示输入数据的批次大小,OUTPUT_SIZE 表示模型输出的大小(通常是分类数)。因此,整个数组可以看作是一个二维数组,其中第 i 行代表第 i 个输入在各个类别上的预测概率。
相关问题
解释代码 static int process(int8_t* input, int* anchor, int grid_h, int grid_w, int height, int width, int stride, std::vector<float>& boxes, std::vector<float>& objProbs, std::vector<int>& classId, float threshold, int32_t zp, float scale) { int validCount = 0; int grid_len = grid_h * grid_w; float thres = unsigmoid(threshold); int8_t thres_i8 = qnt_f32_to_affine(thres, zp, scale); for (int a = 0; a < 3; a++) { for (int i = 0; i < grid_h; i++) { for (int j = 0; j < grid_w; j++) { int8_t box_confidence = input[(PROP_BOX_SIZE * a + 4) * grid_len + i * grid_w + j]; if (box_confidence >= thres_i8) { int offset = (PROP_BOX_SIZE * a) * grid_len + i * grid_w + j; int8_t* in_ptr = input + offset; float box_x = sigmoid(deqnt_affine_to_f32(*in_ptr, zp, scale)) * 2.0 - 0.5; float box_y = sigmoid(deqnt_affine_to_f32(in_ptr[grid_len], zp, scale)) * 2.0 - 0.5; float box_w = sigmoid(deqnt_affine_to_f32(in_ptr[2 * grid_len], zp, scale)) * 2.0; float box_h = sigmoid(deqnt_affine_to_f32(in_ptr[3 * grid_len], zp, scale)) * 2.0; box_x = (box_x + j) * (float)stride; box_y = (box_y + i) * (float)stride; box_w = box_w * box_w * (float)anchor[a * 2]; box_h = box_h * box_h * (float)anchor[a * 2 + 1]; box_x -= (box_w / 2.0); box_y -= (box_h / 2.0); boxes.push_back(box_x); //push_back() 在Vector最后添加一个元素 boxes.push_back(box_y); boxes.push_back(box_w); boxes.push_back(box_h); int8_t maxClassProbs = in_ptr[5 * grid_len]; int maxClassId = 0; for (int k = 1; k < OBJ_CLASS_NUM; ++k) { int8_t prob = in_ptr[(5 + k) * grid_len]; if (prob > maxClassProbs) { maxClassId = k; maxClassProbs = prob; } } objProbs.push_back(sigmoid(deqnt_affine_to_f32(maxClassProbs, zp, scale))); classId.push_back(maxClassId); validCount++; } } } } return validCount; }
这段代码实现了一个目标检测的后处理函数。具体解释如下:
函数参数:
- `input`:输入的 int8_t 类型指针,表示模型输出的特征图。
- `anchor`:包围框的 anchor 锚点,用于计算检测框的位置和大小。
- `grid_h`、`grid_w`:特征图的高度和宽度。
- `height`、`width`:输入图像的高度和宽度。
- `stride`:特征图相对于输入图像的缩放因子。
- `boxes`:存储检测框位置和大小的向量。
- `objProbs`:存储目标置信度的向量。
- `classId`:存储目标类别的向量。
- `threshold`:目标置信度的阈值。
- `zp`、`scale`:量化参数,用于将 int8_t 类型转换为 float 类型。
函数功能:
1. 初始化有效目标计数 `validCount` 为 0。
2. 将阈值 `threshold` 经过反 sigmoid 函数转换为浮点数类型,并使用量化参数将其转换为 int8_t 类型,并赋值给 `thres_i8`。
3. 使用三重循环遍历特征图上的每个格子。
4. 在每个格子上,获取对应的检测框置信度 `box_confidence`,如果其大于等于阈值 `thres_i8`,则表示存在有效目标。
5. 计算检测框的偏移量 `offset`,并通过指针 `in_ptr` 指向对应的检测框信息。
6. 使用量化参数将检测框的位置和大小信息转换为浮点数类型,并进行逆 sigmoid 函数转换。
7. 根据格子的位置、缩放因子和 anchor 锚点,计算得到检测框的真实位置和大小。
8. 将计算得到的检测框位置和大小添加到 `boxes` 向量中。
9. 遍历目标类别,找到具有最大概率的类别,并记录其概率和类别编号。
10. 将目标置信度和类别编号添加到 `objProbs` 和 `classId` 向量中。
11. 增加有效目标计数 `validCount`。
12. 循环结束后,返回有效目标计数。
通过这样的处理过程,可以从模型输出的特征图中提取出有效的目标检测结果,并将检测框的位置、大小、目标置信度和类别编号存储在相应的向量中。
解释代码:static int process(int8_t* input, int* anchor, int grid_h, int grid_w, int height, int width, int stride, std::vector<float>& boxes, std::vector<float>& objProbs, std::vector<int>& classId, float threshold, int32_t zp, float scale) { int validCount = 0; int grid_len = grid_h * grid_w; float thres = unsigmoid(threshold); int8_t thres_i8 = qnt_f32_to_affine(thres, zp, scale); for (int a = 0; a < 3; a++) { for (int i = 0; i < grid_h; i++) { for (int j = 0; j < grid_w; j++) { int8_t box_confidence = input[(PROP_BOX_SIZE * a + 4) * grid_len + i * grid_w + j]; if (box_confidence >= thres_i8) { int offset = (PROP_BOX_SIZE * a) * grid_len + i * grid_w + j; int8_t* in_ptr = input + offset; float box_x = sigmoid(deqnt_affine_to_f32(*in_ptr, zp, scale)) * 2.0 - 0.5; float box_y = sigmoid(deqnt_affine_to_f32(in_ptr[grid_len], zp, scale)) * 2.0 - 0.5; float box_w = sigmoid(deqnt_affine_to_f32(in_ptr[2 * grid_len], zp, scale)) * 2.0; float box_h = sigmoid(deqnt_affine_to_f32(in_ptr[3 * grid_len], zp, scale)) * 2.0; box_x = (box_x + j) * (float)stride; box_y = (box_y + i) * (float)stride; box_w = box_w * box_w * (float)anchor[a * 2]; box_h = box_h * box_h * (float)anchor[a * 2 + 1]; box_x -= (box_w / 2.0); box_y -= (box_h / 2.0); int8_t maxClassProbs = in_ptr[5 * grid_len]; int maxClassId = 0; for (int k = 1; k < OBJ_CLASS_NUM; ++k) { int8_t prob = in_ptr[(5 + k) * grid_len]; if (prob > maxClassProbs) { maxClassId = k; maxClassProbs = prob; } } if (maxClassProbs>thres_i8){ objProbs.push_back(sigmoid(deqnt_affine_to_f32(maxClassProbs, zp, scale))* sigmoid(deqnt_affine_to_f32(box_confidence, zp, scale))); classId.push_back(maxClassId); validCount++; boxes.push_back(box_x); boxes.push_back(box_y); boxes.push_back(box_w); boxes.push_back(box_h); } } } } } return validCount; }
这段代码实现了一个目标检测的后处理函数,与之前的代码相比,增加了对目标置信度和类别概率的判断。具体解释如下:
函数参数和功能与之前的代码相同,不再重复解释。
修改部分:
1. 在检测框的置信度 `box_confidence` 大于等于阈值 `thres_i8` 的条件下,再对目标置信度 `maxClassProbs` 进行判断。
2. 如果 `maxClassProbs` 大于阈值 `thres_i8`,则表示该检测框是一个有效的目标。
3. 将经过 sigmoid 函数转换后的目标置信度和检测框置信度相乘,作为最终的目标置信度。
4. 将最终的目标置信度、类别编号和有效目标计数记录到对应的向量中。
5. 将检测框的位置和大小添加到 `boxes` 向量中。
通过这样的修改,可以实现在进行目标检测后处理时,根据目标置信度和类别概率的阈值进行筛选,将有效的目标的位置、大小、置信度和类别信息存储在相应的向量中。
阅读全文
相关推荐
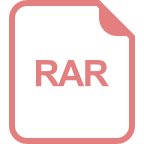
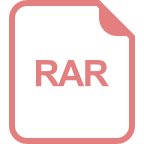









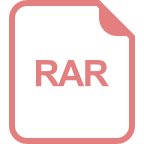
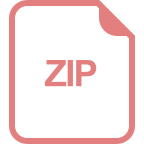
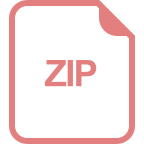