Verilog 一个流水线控制模式的程序
时间: 2024-05-01 19:20:07 浏览: 16
以下是一个简单的基于流水线控制模式的 Verilog 程序示例:
```verilog
module pipeline_control (
input clk, // 时钟信号
input reset, // 复位信号
input start, // 启动信号
output done // 完成信号
);
// 状态定义
parameter IDLE = 0, FETCH = 1, DECODE = 2, EXECUTE = 3, WRITEBACK = 4;
reg [3:0] state, next_state;
// 控制信号定义
reg fetch_enable, decode_enable, execute_enable, writeback_enable;
// 状态寄存器定义
reg [31:0] pc, instruction, opcode, operand1, operand2, result, address;
// 流水线寄存器定义
reg [31:0] fetch_reg, decode_reg, execute_reg, writeback_reg;
always @(posedge clk) begin
if (reset) begin
// 复位时进入 IDLE 状态
state <= IDLE;
fetch_enable <= 0;
decode_enable <= 0;
execute_enable <= 0;
writeback_enable <= 0;
end else begin
// 状态转移逻辑
state <= next_state;
fetch_enable <= (state == FETCH);
decode_enable <= (state == DECODE);
execute_enable <= (state == EXECUTE);
writeback_enable <= (state == WRITEBACK);
end
end
always @(state or fetch_reg or decode_reg or execute_reg or writeback_reg) begin
case (state)
IDLE: begin
// 空闲状态,等待启动信号
next_state = start ? FETCH : IDLE;
end
FETCH: begin
// 取指令阶段
fetch_reg <= $readmemh("program.hex", pc);
next_state = DECODE;
end
DECODE: begin
// 解码阶段
opcode <= fetch_reg[31:24];
operand1 <= fetch_reg[23:16];
operand2 <= fetch_reg[15:8];
address <= fetch_reg[7:0];
next_state = EXECUTE;
end
EXECUTE: begin
// 执行阶段
case (opcode)
0: result <= operand1 + operand2; // 加法
1: result <= operand1 - operand2; // 减法
2: result <= operand1 & operand2; // 按位与
3: result <= operand1 | operand2; // 按位或
4: result <= operand1 ^ operand2; // 按位异或
5: result <= operand1 << operand2; // 逻辑左移
6: result <= operand1 >> operand2; // 逻辑右移
7: result <= $readmemh("memory.hex", address); // 读内存
8: $writememh("memory.hex", address, operand1); // 写内存
default: result <= 0; // 未知指令
endcase
next_state = WRITEBACK;
end
WRITEBACK: begin
// 写回阶段
writeback_reg <= result;
next_state = FETCH;
end
endcase
end
// 完成信号
assign done = (state == IDLE);
endmodule
```
这个程序实现了一个基于 5 个阶段的流水线控制模式,包括取指令、解码、执行、写回和空闲状态。在执行阶段,根据指令类型进行相应的计算或内存读写操作,并将结果写回到流水线寄存器中。然后在写回阶段,将结果写回到内存中。最后,完成信号在 IDLE 状态下被设置为高电平,表示程序已经执行完毕。
相关推荐
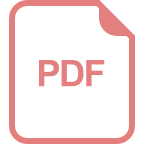
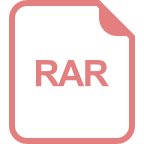
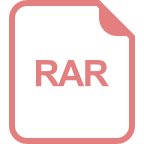














