用Python把a.txt改成b.txt
时间: 2024-02-21 11:57:44 浏览: 56
您可以使用Python的os模块来重命名文件。
以下是示例代码,将a.txt文件重命名为b.txt:
```python
import os
os.rename("a.txt", "b.txt")
```
其中,第一个参数是原始文件名,第二个参数是新文件名。
请注意,如果a.txt和b.txt不在同一目录下,需要提供完整的文件路径。
相关问题
python 1.读取文件,统计里面a出现几次 2.读取a.txt文件,将里面的a全部改成b并写入文件
1. 在Python中,你可以使用内置的`open()`函数来打开和读取文件。如果你想统计'a'出现的次数,可以按照以下步骤操作:
```python
with open('file.txt', 'r') as file:
content = file.read()
count_a = content.count('a')
print(f'"a"出现了{count_a}次')
```
这里我们先读取整个文件内容到变量`content`,然后使用字符串的`count()`方法计算字符'a'出现的次数。
2. 要读取文件并将所有'a'替换为'b',你可以使用`replace()`方法,然后再写回文件:
```python
with open('a.txt', 'r') as read_file:
original_content = read_file.read()
modified_content = original_content.replace('a', 'b')
# 写回新的内容
with open('output.txt', 'w') as write_file:
write_file.write(modified_content)
```
这里首先读取原文件内容,替换了所有的'a'为'b',最后把修改后的内容写入到名为'output.txt'的新文件中。
python 1.读取文件,统计里面a出现几次 2.读取a.txt文件,将里面的a全部改成b并写入文件的程序
1. 要读取文件并在Python中统计某个字符(例如 'a')出现的次数,你可以使用`open()`函数打开文件,然后逐行读取,并利用字符串操作计数。下面是一个简单的例子:
```python
filename = "input.txt" # 更改为你实际的文件名
with open(filename, 'r') as file:
content = file.read() # 读取整个文件内容
count_a = content.count('a') # 统计'a'出现的次数
print(f"'a'出现了{count_a}次.")
```
2. 如果你想读取`a.txt`文件并将所有的 'a' 替换为 'b',然后写回原文件,可以这样做:
```python
# 创建备份,以防原始文件被覆盖
backup_name = filename + '.bak'
if os.path.exists(filename):
shutil.copyfile(filename, backup_name)
with open(filename, 'r+', newline='', encoding='utf-8') as f_in, \
open(filename, 'w', newline='', encoding='utf-8') as f_out:
for line in f_in:
f_out.write(line.replace('a', 'b'))
print("替换完成,新的内容已保存到文件中.")
```
这里假设你的系统有`shutil`库,用于备份文件;如果没有,可以自行添加相应的错误检查和处理。
阅读全文
相关推荐
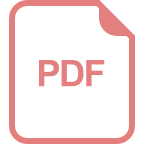
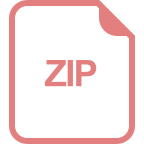


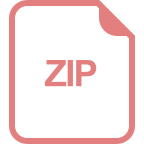
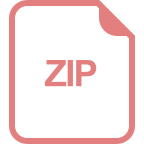
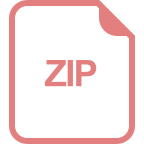
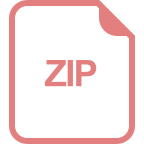
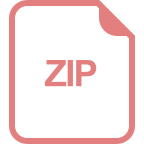
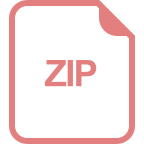
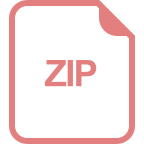
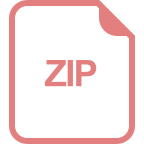
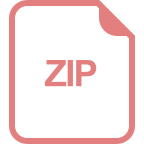
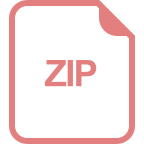
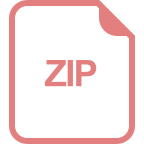
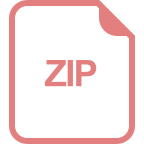