学生信息管理添加功能实现
时间: 2024-02-11 14:01:17 浏览: 61
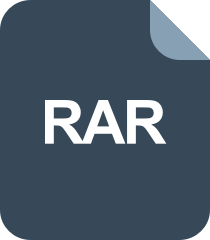
学生信息管理功能
为了实现学生信息管理系统的添加功能,我们需要先定义一个学生类,然后在主函数中创建学生对象并添加到学生列表中。具体步骤如下:
1.定义学生类,包括学号、姓名、性别、年龄、班级等信息。对于小学生和中学生,可以在学生类中添加相应的成绩和其他信息。
```C++
class Student {
public:
string id; // 学号
string name; // 姓名
string gender; // 性别
int age; // 年龄
string grade; // 班级
// 小学生信息
int chineseScore; // 语文成绩
int mathScore; // 数学成绩
int englishScore; // 英语成绩
// 中学生信息
int geographyScore; // 地理成绩
int historyScore; // 历史成绩
string address; // 家庭住址
};
```
2.在主函数中创建学生列表,并实现添加学生的功能。可以通过用户输入学生信息来创建学生对象,并将其添加到学生列表中。
```C++
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<Student> students; // 学生列表
int n; // 添加学生的数量
cout << "请输入要添加的学生数量:";
cin >> n;
for (int i = 0; i < n; i++) {
Student student;
cout << "请输入第" << i+1 << "个学生的信息:" << endl;
cout << "学号:";
cin >> student.id;
cout << "姓名:";
cin >> student.name;
cout << "性别:";
cin >> student.gender;
cout << "年龄:";
cin >> student.age;
cout << "班级:";
cin >> student.grade;
// 小学生信息
if (student.grade.find("小学") != string::npos) {
cout << "语文成绩:";
cin >> student.chineseScore;
cout << "数学成绩:";
cin >> student.mathScore;
cout << "英语成绩:";
cin >> student.englishScore;
}
// 中学生信息
else if (student.grade.find("中学") != string::npos) {
cout << "语文成绩:";
cin >> student.chineseScore;
cout << "数学成绩:";
cin >> student.mathScore;
cout << "英语成绩:";
cin >> student.englishScore;
cout << "地理成绩:";
cin >> student.geographyScore;
cout << "历史成绩:";
cin >> student.historyScore;
cout << "家庭住址:";
cin >> student.address;
}
students.push_back(student); // 添加学生到学生列表中
}
cout << "添加成功!" << endl;
return 0;
}
```
3.运行程序,输入要添加的学生数量和每个学生的信息,即可实现学生信息的添加功能。
阅读全文
相关推荐
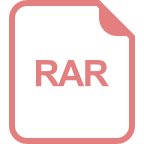
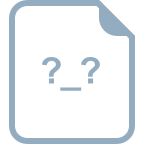
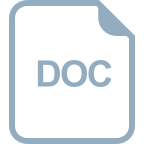
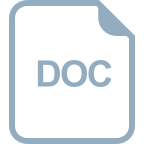
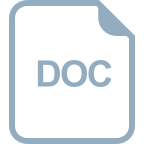
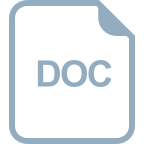
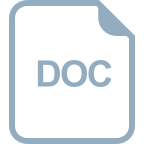
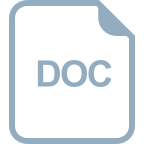
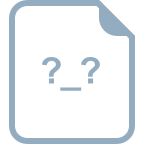
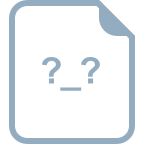
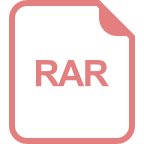
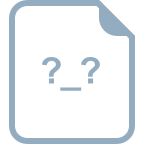
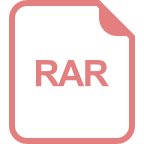
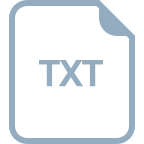
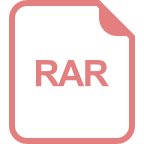
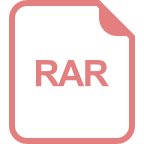