统计字符串中不同种类的字符个数: 本题目要求读入一个字符串,统计字符串中字母、数字、空格、其它字符的个数。 输入格式: 输入一行由任意字符组成的字符串。 输出格式: 统计字符串中字母、数字、空格、其它字符的个数。
时间: 2023-05-27 10:07:56 浏览: 136
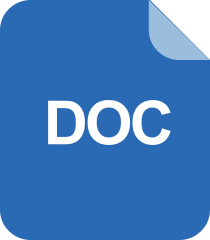
输入一行字符,分别统计出其中英文字母、空格、数字和其它字符的个数
算法1:
循环遍历字符串中的每一个字符,判断其类型并计数。
C++ 代码
#include <iostream>
using namespace std;
int main() {
string s;
getline(cin, s); // 读入一行字符串
int letter = 0, digit = 0, space = 0, other = 0;
for (int i = 0; i < s.length(); i++) {
char c = s[i];
if (c >= 'a' && c <= 'z' || c >= 'A' && c <= 'Z') {
letter++;
} else if (c >= '0' && c <= '9') {
digit++;
} else if (c == ' ') {
space++;
} else {
other++;
}
}
cout << "letter:" << letter << endl;
cout << "digit:" << digit << endl;
cout << "space:" << space << endl;
cout << "other:" << other << endl;
return 0;
}
算法2:
使用STL中的isalpha、isdigit、isspace函数判断字符类型。
C++ 代码
#include <iostream>
#include <cctype> // 包含isalpha、isdigit、isspace函数
using namespace std;
int main() {
string s;
getline(cin, s); // 读入一行字符串
int letter = 0, digit = 0, space = 0, other = 0;
for (int i = 0; i < s.length(); i++) {
char c = s[i];
if (isalpha(c)) {
letter++;
} else if (isdigit(c)) {
digit++;
} else if (isspace(c)) {
space++;
} else {
other++;
}
}
cout << "letter:" << letter << endl;
cout << "digit:" << digit << endl;
cout << "space:" << space << endl;
cout << "other:" << other << endl;
return 0;
}
算法3:
使用map来保存每种字符出现的次数。
C++ 代码
#include <iostream>
#include <map>
using namespace std;
int main() {
string s;
getline(cin, s); // 读入一行字符串
map<char, int> cnt;
for (int i = 0; i < s.length(); i++) {
char c = s[i];
if (isalpha(c)) {
cnt['a']++;
} else if (isdigit(c)) {
cnt['d']++;
} else if (isspace(c)) {
cnt['s']++;
} else {
cnt['o']++;
}
}
cout << "letter:" << cnt['a'] << endl;
cout << "digit:" << cnt['d'] << endl;
cout << "space:" << cnt['s'] << endl;
cout << "other:" << cnt['o'] << endl;
return 0;
}
阅读全文
相关推荐














