捕食者猎物模型python函数
时间: 2023-10-29 21:14:42 浏览: 130
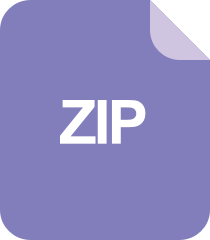
RSwarm:强化学习的猎物模型
以下是一个简单的捕食者猎物模型的Python函数:
```python
def predator_prey_model(x, y, a, b, c, d, h):
"""
Predator-Prey Model function
x: prey population
y: predator population
a: prey growth rate
b: predator mortality rate
c: conversion efficiency
d: predator growth rate
h: predator interference factor
"""
dx_dt = a * x - b * x * y
dy_dt = c * b * x * y - h * d * y
return dx_dt, dy_dt
```
这个函数接受6个参数:猎物种群(x),捕食者种群(y),猎物增长率(a),捕食者死亡率(b),转化效率(c),捕食者增长率(d)和捕食者干扰因子(h)。函数返回一个元组,包含猎物和捕食者种群的变化率(dx/dt和dy/dt)。
使用这个函数,我们可以实现一个简单的模拟程序,来预测猎物和捕食者种群随时间的变化。例如:
```python
import numpy as np
from scipy.integrate import odeint
import matplotlib.pyplot as plt
# Set parameters
a = 1.2
b = 0.6
c = 0.8
d = 0.3
h = 0.2
# Set initial conditions
x0 = 10
y0 = 5
# Set time range
t = np.linspace(0, 20, 1000)
# Solve the ODE system
y = odeint(predator_prey_model, (x0, y0), t, args=(a, b, c, d, h))
# Plot the results
plt.plot(t, y[:, 0], label="Prey")
plt.plot(t, y[:, 1], label="Predator")
plt.xlabel("Time")
plt.ylabel("Population")
plt.legend()
plt.show()
```
这个程序使用SciPy库中的odeint函数,数值求解了ODE系统,并使用matplotlib库绘制了猎物和捕食者种群随时间的变化图。在这个例子中,猎物种群增长率高于捕食者死亡率,因此猎物种群增加,捕食者种群也随之增加,但增长速度比猎物慢,最终达到一个稳定状态。
阅读全文
相关推荐
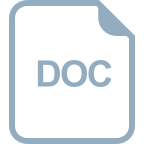
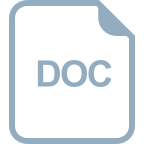




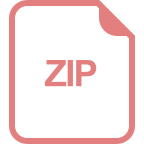
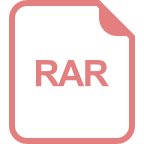
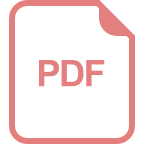





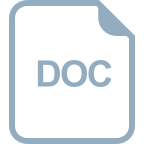
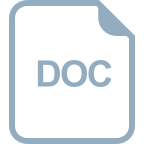
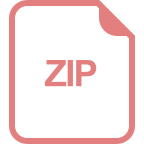
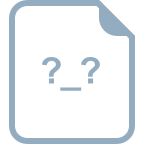