酒店预定系统中保存了大量的客户评价信息,为了更好的服务客户,我们需要对评价信息进行分析。每条评价信息由一个int类型的ID和字符串类型的内容构成。请根据提供的评价ID和内容,设计对搜索单词的倒排索引,实现根据输入要查找的单词(区分大小写),输出包含单词的评价ID。 示例1 输入:[1, 5, 4, 9],["Great", "Beautiful", "you are young", "Great hotel"],"Great" 返回值:[1,9]
时间: 2023-02-08 15:42:00 浏览: 72
为了实现倒排索引,可以创建一个 HashMap,其中键为要查找的单词,值为包含该单词的评价ID的列表。
首先,将评价ID和内容的对应关系存储到 HashMap 中。然后,对于每条评价信息,将内容拆分成单词数组,并遍历数组中的每个单词。如果 HashMap 中不存在当前单词,则创建一个新的键值对,并将评价ID添加到值中。如果 HashMap 中已经存在当前单词,则在对应的值中添加评价ID。
最后,可以通过查询 HashMap 来获取包含指定单词的评价ID的列表。
以下是一个使用 Java 实现的示例:
```
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class InvertedIndex {
public static List<Integer> search(int[] ids, String[] contents, String word) {
Map<String, List<Integer>> index = buildIndex(ids, contents);
return index.get(word);
}
private static Map<String, List<Integer>> buildIndex(int[] ids, String[] contents) {
Map<String, List<Integer>> index = new HashMap<>();
for (int i = 0; i < ids.length; i++) {
int id = ids[i];
String content = contents[i];
String[] words = content.split("\\s+");
for (String word : words) {
List<Integer> idsList = index.get(word);
if (idsList == null) {
idsList = new ArrayList<>();
index.put(word, idsList);
}
idsList.add(id);
}
}
return index;
}
}
```
使用方式:
```
int[] ids = {1, 5, 4, 9};
String[] contents = {"Great", "Beautiful", "you are young", "Great hotel"};
List<Integer> result = Inverted
相关推荐
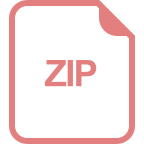
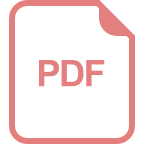
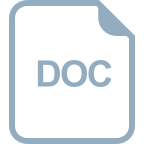














