在UCI数据集中寻找一个二分类数据集,编程实现决策树分类模型;在划分时可以使用信息增益、增益率或基尼指数进行选择划分属性。要求画出图形。
时间: 2024-03-30 14:36:46 浏览: 17
好的,我明白了你的问题。首先,我需要知道你要使用哪个UCI数据集来进行分类。然后,我可以为你提供一些Python代码来实现决策树分类模型,并且使用信息增益、增益率或基尼指数进行选择划分属性。
假设你选择了UCI的Breast Cancer Wisconsin (Diagnostic) Data Set。那么你可以从下面的链接中下载数据集:
https://archive.ics.uci.edu/ml/datasets/breast+cancer+wisconsin+(diagnostic)
然后,你可以使用下面的Python代码来实现决策树分类模型,并且使用信息增益、增益率或基尼指数进行选择划分属性:
```
import pandas as pd
from sklearn.tree import DecisionTreeClassifier
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
from sklearn.tree import export_graphviz
import graphviz
# Load the dataset
url = 'https://archive.ics.uci.edu/ml/machine-learning-databases/breast-cancer-wisconsin/wdbc.data'
df = pd.read_csv(url, header=None)
# Split the dataset into features and target
X = df.iloc[:, 2:].values
y = df.iloc[:, 1].values
# Split the dataset into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Create the decision tree classifier
clf = DecisionTreeClassifier(criterion='entropy', random_state=42)
# Fit the decision tree classifier to the training data
clf.fit(X_train, y_train)
# Use the decision tree classifier to make predictions on the testing data
y_pred = clf.predict(X_test)
# Calculate the accuracy of the decision tree classifier
accuracy = accuracy_score(y_test, y_pred)
print('Accuracy:', accuracy)
# Export the decision tree as a Graphviz file
dot_data = export_graphviz(clf, out_file=None,
feature_names=df.columns[2:],
class_names=df.columns[1],
filled=True, rounded=True,
special_characters=True)
graph = graphviz.Source(dot_data)
graph.render('breast_cancer_diagnostic_tree')
```
这段代码会将数据集加载到Pandas DataFrame中,并将其拆分为特征和目标。然后,它将数据集拆分为训练集和测试集,并使用信息增益作为划分准则来创建决策树分类器。最后,它将决策树以Graphviz格式导出为一个文件,并将其保存为“breast_cancer_diagnostic_tree.pdf”文件。
你可以使用Graphviz软件来查看决策树。如果你没有安装Graphviz,你可以使用下面的代码来在Jupyter Notebook中显示决策树:
```
from IPython.display import Image
Image(filename='breast_cancer_diagnostic_tree.png')
```
这段代码会在Jupyter Notebook中显示决策树的图形。
相关推荐
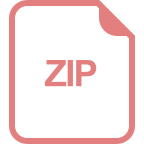














