IndexError: too many indices for array: array is 3-dimensional, but 4 were indexed
时间: 2024-03-06 15:47:28 浏览: 81
这个错误通常是因为在获取卷积层输出时,使用的是四维的索引,但是卷积层的输出是三维的。你可以尝试修改代码,将 `conv_output[0, :, :, i]` 修改为 `conv_output[0, :, i]`,或者使用 `np.squeeze()` 函数来去掉维度为 1 的维度。以下是修改后的代码:
```
from keras import backend as K
import numpy as np
# 定义一个函数,用于获取卷积层的输出
get_conv_output = K.function([model.layers[0].input], [model.layers[0].output])
# 获取卷积层的输出
conv_output = get_conv_output([X])[0]
# 将输出可视化
plt.figure(figsize=(10, 10))
for i in range(32):
plt.subplot(4, 8, i+1)
plt.imshow(np.squeeze(conv_output[0, :, :, i]), cmap='gray')
plt.show()
```
这段代码中,使用了 `np.squeeze()` 函数将维度为 1 的维度去掉,然后再将卷积层输出可视化。
相关问题
IndexError: too many indices for array: array is 2-dimensional, but 3 were indexed
This error message indicates that you are trying to access an element in a NumPy array using too many indices.
For example, suppose you have a 2-dimensional array (matrix) with shape (3, 3), and you try to access an element using three indices like this:
```python
import numpy as np
arr = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
elem = arr[1, 2, 0] # this will raise an IndexError
```
In this case, the `arr[1, 2, 0]` statement is trying to access an element at row 1, column 2, and depth 0 (which doesn't exist because the array is only 2-dimensional).
To fix this error, make sure you are using the correct number of indices for your array. For a 2-dimensional array, you should only use two indices (one for the row and one for the column).
IndexError: too many indices for array: array is 1-dimensional, but 3 were indexed
这个错误是由于对一个一维数组使用了超过其维度的索引导致的。在你提供的引用中,报错是因为对一个一维数组使用了两个索引。在你提供的引用中,代码尝试将一个二维数组改变为三维数组,但是使用了三个索引。
以下是两个例子来解释这个错误:
1. 对一维数组使用超过其维度的索引:
```python
import numpy as np
a = np.array([1, 2, 3, 4, 5])
print(a[0]) # 输出:1
print(a[1]) # 输出:2
print(a[2]) # 输出:3
print(a[3]) # 输出:4
print(a[4]) # 输出:5
print(a[5]) # 报错:IndexError: too many indices for array: array is 1-dimensional, but 2 were indexed
```
2. 将二维数组改变为三维数组时使用超过其维度的索引:
```python
import numpy as np
a = np.array([[1, 2, 3], [4, 5, 6]])
print(a.shape) # 输出:(2, 3)
b = a.reshape(-1, 1)
print(b.shape) # 输出:(6, 1)
c = a.reshape(1, -1)
print(c.shape) # 输出:(1, 6)
print(a[0, 0]) # 输出:1
print(a[0, 1]) # 输出:2
print(a[0, 2]) # 输出:3
print(a[1, 0]) # 输出:4
print(a[1, 1]) # 输出:5
print(a[1, 2]) # 输出:6
print(a[0, 0, 0]) # 报错:IndexError: too many indices for array: array is 1-dimensional, but 3 were indexed
```
阅读全文
相关推荐
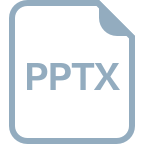
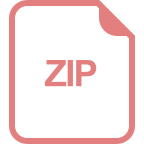


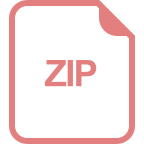
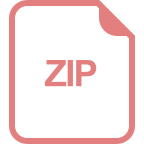
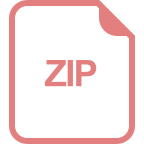
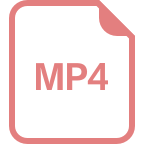
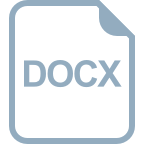
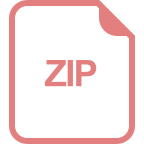
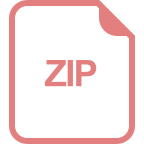
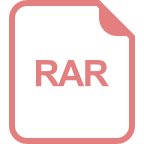
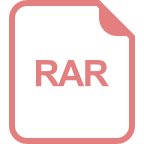
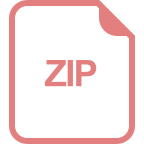