设计关于Android的鲜花商店代码
时间: 2023-10-11 21:09:39 浏览: 107
好的,下面是一个简单的Android鲜花商店的代码示例,仅供参考:
1. activity_main.xml(商店首页)
```
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<!-- 标题栏 -->
<include layout="@layout/layout_title_bar" />
<!-- 商品列表 -->
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/rv_product_list"
android:layout_width="match_parent"
android:layout_height="match_parent" />
</LinearLayout>
```
2. layout_title_bar.xml(标题栏布局)
```
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<ImageView
android:id="@+id/iv_back"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/ic_back" />
<TextView
android:id="@+id/tv_title"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="鲜花商店"
android:textColor="@color/black"
android:textSize="18sp" />
<ImageView
android:id="@+id/iv_cart"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/ic_cart" />
</LinearLayout>
```
3. Product.java(商品实体类)
```
public class Product {
private int id;
private String name;
private String imageUrl;
private double price;
public Product(int id, String name, String imageUrl, double price) {
this.id = id;
this.name = name;
this.imageUrl = imageUrl;
this.price = price;
}
// 省略getter和setter方法
}
```
4. ProductAdapter.java(商品列表适配器)
```
public class ProductAdapter extends RecyclerView.Adapter<ProductAdapter.ViewHolder> {
private List<Product> productList;
private OnItemClickListener onItemClickListener;
public ProductAdapter(List<Product> productList) {
this.productList = productList;
}
@NonNull
@Override
public ViewHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) {
View view = LayoutInflater.from(parent.getContext()).inflate(R.layout.item_product, parent, false);
return new ViewHolder(view);
}
@Override
public void onBindViewHolder(@NonNull ViewHolder holder, int position) {
Product product = productList.get(position);
holder.tvName.setText(product.getName());
holder.tvPrice.setText(String.format(Locale.getDefault(), "%.2f", product.getPrice()));
Glide.with(holder.itemView.getContext()).load(product.getImageUrl()).into(holder.ivImage);
holder.itemView.setOnClickListener(v -> {
if (onItemClickListener != null) {
onItemClickListener.onItemClick(product);
}
});
}
@Override
public int getItemCount() {
return productList.size();
}
static class ViewHolder extends RecyclerView.ViewHolder {
ImageView ivImage;
TextView tvName;
TextView tvPrice;
public ViewHolder(@NonNull View itemView) {
super(itemView);
ivImage = itemView.findViewById(R.id.iv_product_image);
tvName = itemView.findViewById(R.id.tv_product_name);
tvPrice = itemView.findViewById(R.id.tv_product_price);
}
}
public interface OnItemClickListener {
void onItemClick(Product product);
}
public void setOnItemClickListener(OnItemClickListener onItemClickListener) {
this.onItemClickListener = onItemClickListener;
}
}
```
5. item_product.xml(商品列表项布局)
```
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:padding="16dp">
<ImageView
android:id="@+id/iv_product_image"
android:layout_width="80dp"
android:layout_height="80dp"
android:scaleType="centerCrop" />
<LinearLayout
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:orientation="vertical"
android:layout_marginStart="16dp">
<TextView
android:id="@+id/tv_product_name"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textStyle="bold"
android:textSize="16sp" />
<TextView
android:id="@+id/tv_product_price"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textColor="@color/colorAccent"
android:textSize="14sp" />
</LinearLayout>
</LinearLayout>
```
以上是一个简单的Android鲜花商店的代码示例,实现了商店首页的商品列表展示功能。当然,这只是一个开始,还需要进一步完善其他功能模块的代码。
阅读全文
相关推荐






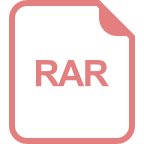









