Android kotlin 截屏
时间: 2024-12-26 20:27:29 浏览: 4
### 如何在 Android 应用中使用 Kotlin 实现截屏功能
要在 Android 中通过 Kotlin 获取屏幕截图并保存到设备存储,可以按照如下方法:
#### 创建权限请求
为了能够读取和写入外部存储,在 `AndroidManifest.xml` 文件中声明必要的权限[^1]:
```xml
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE"/>
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/>
```
#### 编写获取屏幕截图的方法
下面是一个简单的函数来捕获当前活动窗口的内容,并将其转换成位图图像:
```kotlin
fun takeScreenshot(context: Context, view: View): Bitmap {
val bitmap = Bitmap.createBitmap(view.width, view.height, Bitmap.Config.ARGB_8888)
val canvas = Canvas(bitmap)
view.draw(canvas)
return bitmap
}
```
#### 将位图保存至文件系统
此辅助函数负责将生成的位图对象保存为JPEG格式图片文件:
```kotlin
@Throws(IOException::class)
fun saveImageToExternalStorage(bitmap: Bitmap, filename: String) : File? {
var fos: FileOutputStream? = null
try {
MediaStore.Images.Media.insertImage(
context.contentResolver,
bitmap,
"$filename.jpg",
"screenshot"
)
val file = File(Environment.getExternalStorageDirectory(), "${filename}.jpg")
fos = FileOutputStream(file)
if (bitmap.compress(Bitmap.CompressFormat.JPEG, 100, fos)) {
fos.flush()
fos.close()
// Notify gallery app that there is a new image available so it can be seen immediately.
context.sendBroadcast(Intent(Intent.ACTION_MEDIA_SCANNER_SCAN_FILE, Uri.fromFile(file)))
return file
}
} catch(e: Exception){
e.printStackTrace()
} finally{
fos?.close()
}
return null
}
```
#### 调用上述两个函数以完成整个过程
当希望触发一次新的屏幕抓取操作时,可以在适当的地方调用这两个函数组合而成的新函数:
```kotlin
private fun captureAndSaveScreen() {
val rootView = findViewById<View>(android.R.id.content).rootView
val screenshotBmp = takeScreenshot(this, rootView)
try {
val savedFile = saveImageToExternalStorage(screenshotBmp, System.currentTimeMillis().toString())
Toast.makeText(applicationContext,"Saved:$savedFile",Toast.LENGTH_LONG).show()
}catch(ex:Exception){
ex.printStackTrace()
Toast.makeText(applicationContext,"Failed to Save Screenshot!",Toast.LENGTH_SHORT).show()
}
}
```
阅读全文
相关推荐
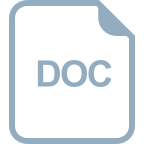
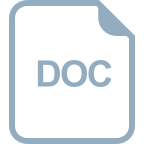
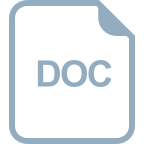
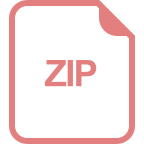
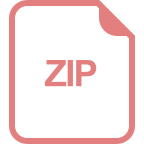
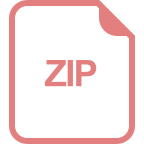
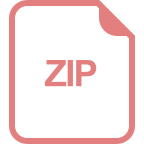


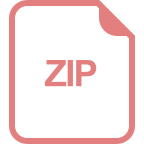
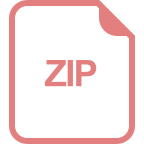
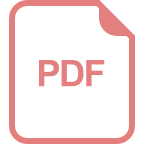
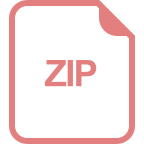
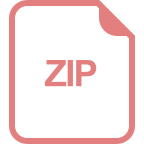
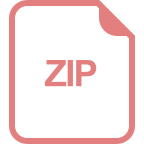
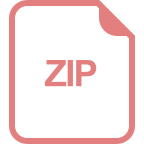
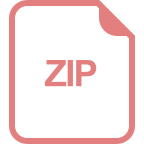
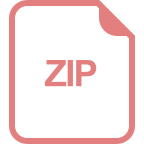
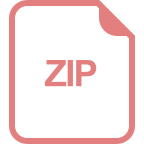