写一个c++多线程回调函数的案例,主线程可注册
时间: 2024-09-14 07:17:31 浏览: 62
在C++中,我们可以使用`std::thread`创建多线程,并通过回调函数(也称为委托函数)来传递任务并处理结果。这里有一个简单的例子,展示了如何创建一个多线程并设置一个回调函数:
```cpp
#include <iostream>
#include <thread>
#include <function>
// 定义回调函数类型
using CallbackType = void(*)(int result);
// 线程完成后的回调函数示例
void callback(int result) {
std::cout << "Thread finished with result: " << result << std::endl;
}
// 主线程要执行的任务,这里是一个模拟的耗时操作
void performTask(CallbackType callback) {
int result = calculateExpensiveOperation(); // 模拟计算操作
callback(result);
}
// 模拟昂贵的计算操作
int calculateExpensiveOperation() {
std::this_thread::sleep_for(std::chrono::seconds(5));
return 42;
}
int main() {
// 创建一个线程并传入回调函数
std::thread workerThread(performTask, std::ref(callback));
// 主线程继续执行其他任务
std::cout << "Main thread starts" << std::endl;
// 等待线程结束
workerThread.join();
std::cout << "Main thread ended" << std::endl;
return 0;
}
```
在这个例子中,`main`线程启动了一个新线程`workerThread`,该线程接受一个`performTask`的引用作为参数,`performTask`会执行一段模拟的耗时操作,完成后通过`callback`将结果传递回主线程。
阅读全文
相关推荐
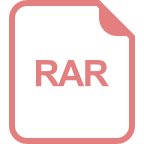

















