valuation Pretrained models from the model zoo can be evaluated using the `eval.py` script. For example, the model on cifar-10 can be evaluated as follows: ```shell python eval.py --config_exp configs/scan/scan_cifar10.yml --model $MODEL_PATH ``` Visualizing the prototype images is easily done by setting the `--visualize_prototypes` flag. For example on cifar-10: <p align="center"> <img src="images/prototypes_cifar10.jpg" width="600" /> </p> Similarly, you might want to have a look at the clusters found on ImageNet (as shown at the top). First download the model (link in table above) and then execute the following command: ```shell python eval.py --config_exp configs/scan/wjd_eval.yml --model $MODEL_PATH_IMAGENET ```,这段话说了啥,能解释一下吗
时间: 2024-02-25 11:56:07 浏览: 19
这段话是在介绍如何使用预训练模型进行评估和可视化。预训练模型可以从模型库中获取。以CIFAR-10为例,可以使用以下命令进行评估:```shell python eval.py --config_exp configs/scan/scan_cifar10.yml --model $MODEL_PATH```。如果需要可视化原型图像,只需设置`--visualize_prototypes`标志即可。例如,在CIFAR-10上,可以使用以下命令进行可视化:```shell python eval.py --config_exp configs/scan/wjd_eval.yml --model $MODEL_PATH_IMAGENET```。同样,如果需要查看在ImageNet上找到的聚类结果,则需要先下载该模型(链接在上述表格中),然后执行以上命令。
相关问题
中文回答What is Contingent Valuation? What may cause the bias of Contingent Valuation?
条件估价(Contingent Valuation)是一种用于评估某种商品或服务的非市场价值的方法。它通过调查人们愿意支付多少钱来获得该商品或服务,从而评估该商品或服务的价值。
然而,条件估价也可能存在偏差。以下是可能导致条件估价偏差的一些因素:
1. 社交偏好:受访者可能会在问卷调查中报告比他们实际愿意支付更高的价格,以遵循社会期望或期望他人的行为。
2. 定向反应:受访者可能会在问卷调查中报告他们认为理应支付的价格,而不是他们实际愿意支付的价格。
3. 信息偏差:受访者可能会对商品或服务的价值缺乏了解,导致他们的应答偏差。
4. 问卷设计偏差:问卷设计不当可能会导致偏差,例如问题的顺序或问法可能会影响受访者的回答。
因此,在使用条件估价方法时,需要认真考虑这些因素,并采取适当的纠正方法来避免或减少偏差。
详细举例This code represents a class named "PropertyManagementCompany" which manages a portfolio of real estate properties. The class has a name, liquidity and a set of properties that it manages. The liquidity represents the amount of money the company has available to purchase new properties or sell existing ones. The class has two constructors, one that creates an empty portfolio and sets the liquidity to zero, and another that takes a list of properties and adds them to the portfolio. Both constructors also require the company name and liquidity as parameters. The class has two methods to buy and sell real estate properties. The "buyProperty" method takes a property and a purchase price as parameters and adds the property to the portfolio if the company has enough liquidity to buy it. The "sellProperty" method takes a property and removes it from the portfolio while adding the property's current valuation to the company's liquidity. The class also has getters for the name, liquidity, and portfolio. The "checkName" method is a private helper method that checks if the company name is empty and throws an exception if it is. Overall, this code uses encapsulation to hide the implementation details of the PropertyManagementCompany class. The class has private fields that can only be accessed through its public methods. This approach makes the code more modular and easier to maintain.
```python
class PropertyManagementCompany:
def __init__(self, name, liquidity, properties=None):
self.checkName(name)
self.name = name
self.liquidity = liquidity
if properties is None:
self.portfolio = []
else:
self.portfolio = properties
def buyProperty(self, property, purchasePrice):
if self.liquidity >= purchasePrice:
self.portfolio.append(property)
self.liquidity -= purchasePrice
def sellProperty(self, property):
if property in self.portfolio:
self.portfolio.remove(property)
self.liquidity += property.currentValuation
def getName(self):
return self.name
def getLiquidity(self):
return self.liquidity
def getPortfolio(self):
return self.portfolio
def checkName(self, name):
if name == '':
raise ValueError('Company name cannot be empty.')
```
For example, we can create a new PropertyManagementCompany as follows:
```python
properties = [Property('123 Main St.', 100000), Property('456 Oak Ave.', 200000)]
company = PropertyManagementCompany('ABC Company', 500000, properties)
```
This creates a company named "ABC Company" with $500,000 liquidity and a portfolio containing two properties. We can then buy a new property and sell an existing property using the `buyProperty` and `sellProperty` methods:
```python
newProperty = Property('789 Elm St.', 150000)
company.buyProperty(newProperty, 150000)
propertyToSell = properties[0]
company.sellProperty(propertyToSell)
```
This would add the new property to the portfolio and remove the first property from the portfolio while adding its current valuation to the company's liquidity.
相关推荐
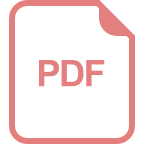
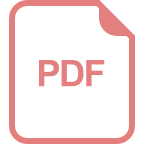













