如何利用python实现注意力机制
时间: 2023-12-16 11:20:48 浏览: 163
要实现注意力机制,需要使用以下步骤:
1. 安装必要的Python库,如TensorFlow和NumPy。
2. 准备输入数据,并将其编码为向量形式。
3. 定义注意力机制的模型架构,包括编码器和解码器。编码器将输入数据映射到隐藏表示形式,而解码器根据这些隐藏表示形式生成输出。
4. 实现注意力机制的正向传递(forward pass),在解码器中使用注意力向量,以根据当前隐藏表示形式计算每个输入位置的权重。
5. 训练模型,使用误差函数对模型进行优化,并用反向传播算法更新模型参数。
以下是一个实现注意力机制的代码示例:
```python
import tensorflow as tf
import numpy as np
class Attention(tf.keras.layers.Layer):
def __init__(self):
super(Attention, self).__init__()
def build(self, input_shape):
self.W1 = self.add_weight(name="W1", shape=(input_shape[-1], input_shape[-1]), initializer="random_normal", trainable=True)
self.W2 = self.add_weight(name="W2", shape=(input_shape[-1], input_shape[-1]), initializer="random_normal", trainable=True)
self.V = self.add_weight(name="V", shape=(input_shape[-1], 1), initializer="random_normal", trainable=True)
def call(self, inputs):
query, values = inputs[0], inputs[1]
query_with_time_axis = tf.expand_dims(query, 1)
score = tf.matmul(tf.nn.tanh(tf.matmul(query_with_time_axis, self.W1) + tf.matmul(values, self.W2)), self.V)
attention_weights = tf.nn.softmax(score, axis=1)
context_vector = attention_weights * values
context_vector = tf.reduce_sum(context_vector, axis=1)
return context_vector, attention_weights
```
此代码段实现了自注意力机制。需要注意的是,Attention类是tf.keras.layers.Layer的子类,因此可以将其用作Keras模型中的层。在这个例子中,attention_weights是每个输入向量的注意力得分,context_vector是加权平均值。
阅读全文
相关推荐
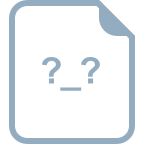
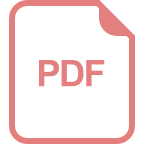
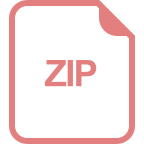
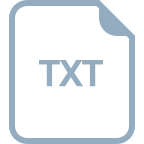
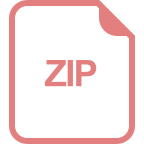
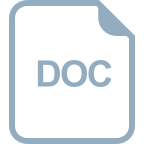
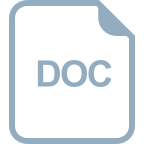
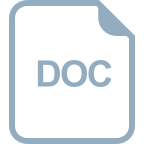
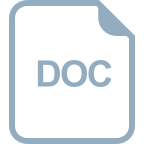
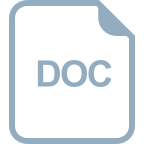
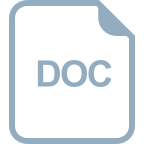






