JDK 17 新功能示例代码
时间: 2023-07-23 16:01:23 浏览: 183
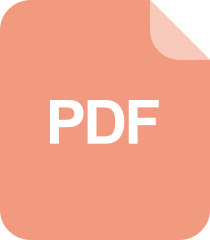
Java17新特性详解含示例代码(值得珍藏)
以下是一些 JDK 17 新功能的示例代码:
1. Sealed Classes:
```java
public abstract sealed class Shape permits Circle, Square {
// ...
}
public final class Circle extends Shape {
// ...
}
public final class Square extends Shape {
// ...
}
```
2. Pattern Matching for switch:
```java
public String getDayOfWeek(int day) {
return switch (day) {
case 1 -> "Monday";
case 2 -> "Tuesday";
case 3 -> "Wednesday";
case 4 -> "Thursday";
case 5 -> "Friday";
case 6, 7 -> "Weekend";
default -> throw new IllegalArgumentException("Invalid day");
};
}
```
3. Strong encapsulation of JDK internals:
```java
import jdk.internal.util.ReflectionUtil;
public class InternalAPIExample {
public static void main(String[] args) {
ReflectionUtil.invokeMethod(/* internal API method */);
}
}
```
4. Foreign Function & Memory API:
```java
import jdk.incubator.foreign.MemorySegment;
import jdk.incubator.foreign.ResourceScope;
public class MemoryExample {
public static void main(String[] args) {
try (ResourceScope scope = ResourceScope.newConfinedScope()) {
MemorySegment segment = MemorySegment.allocateNative(1024, scope);
// ...
}
}
}
```
这些示例代码展示了 JDK 17 中一些新功能的用法。请注意,某些示例可能需要引入特定的包或模块。确保你的项目配置正确以使用这些功能。
阅读全文
相关推荐
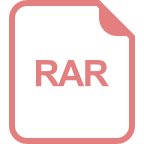
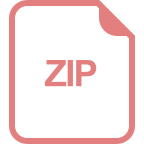
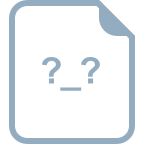
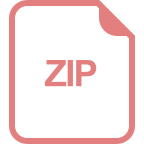
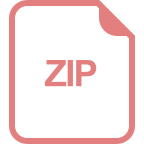
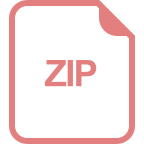
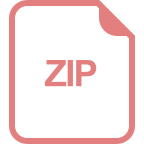
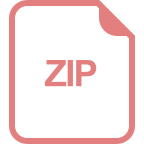
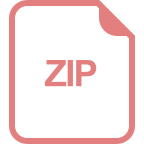
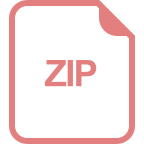
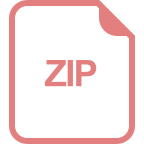
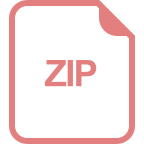
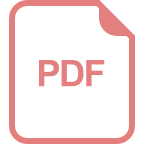
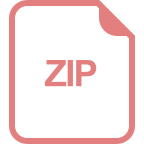