写一个 stm32ct86 运用四个编码电机的程序
时间: 2024-12-13 21:19:21 浏览: 2
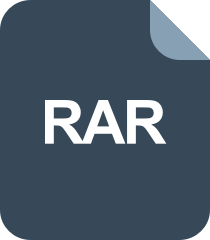
stm32f405编码电机程序及原理图.rar

STM32CT86是一种针对微控制器的软件框架,通常用于基于STM32系列芯片的嵌入式系统项目,但它并非实际的产品型号。不过,假设你有一个基于STM32F4/F7等支持CAN通信或者步进电机控制功能的硬件平台,并想控制四个编码电机,我们可以大概给出一个通用的步骤:
首先,确保你已经配置了STM32的GPIO作为电机的输入/输出以及连接编码器的信号线。然后你可以编写如下的伪代码概述:
```c
#include "stm32f4xx.h"
#include "stm32_can.h"
#include "encoder_driver.h"
// 定义电机结构体和全局变量
typedef struct {
GPIO_TypeDef* gpio;
uint16_t step_pin; // 步进脉冲
uint16_t dir_pin; // 方向控制
Encoder encoder;
} Motor;
Motor motors[4];
void setup_motors() {
for (int i = 0; i < 4; i++) {
// 初始化GPIO和编码器驱动
motors[i].gpio = ...; // GPIO初始化
motors[i].step_pin = ...;
motors[i].dir_pin = ...;
encoder_init(&motors[i].encoder, ...);
}
}
void motor_step(int motor_id, int direction) {
HAL_GPIO_WritePin(motors[motor_id].dir_pin, direction ? GPIO_PIN_SET : GPIO_PIN_RESET);
HAL_Delay(1); // 脉冲间隔时间
HAL_GPIO_WritePin(motors[motor_id].step_pin, GPIO_PIN_SET);
encoder_update(&motors[motor_id].encoder);
HAL_Delay(1);
HAL_GPIO_WritePin(motors[motor_id].step_pin, GPIO_PIN_RESET);
}
void can_send_motor_command(CAN_HandleTypeDef *hcan, int motor_id, int position) {
CAN_Message message;
... // 构造并发送包含电机ID和目标位置的数据包
HAL_CAN_Transmit(hcan, &message, 1, 1000);
}
int main(void) {
STM32CanInit();
setup_motors();
while (1) {
CAN_Message received_message;
if (HAL_CAN_Receive(&hcan, &received_message, 1, 100)) {
int motor_id = received_message.id >> X; // 解析CAN ID获取电机ID
int target_position = received_message.data[0] + received_message.data[1]*256; // 解码数据到目标位置
motor_step(motor_id, target_position > motors[motor_id].current_encoder_pos ? 1 : 0); // 控制电机转向
}
}
}
```
在这个示例中,我们假设了通过CAN总线接收来自上位机的目标电机位置信息。实际操作中,你需要根据具体的硬件配置、库函数和通信协议调整细节。
阅读全文
相关推荐
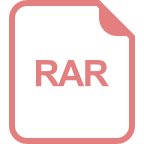
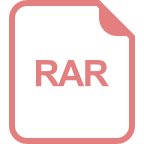
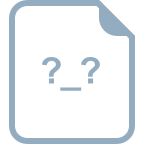
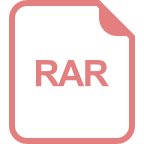
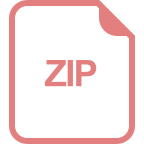
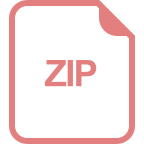
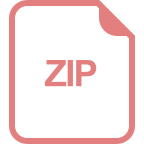
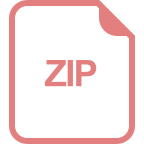
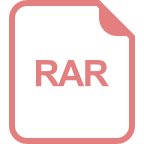
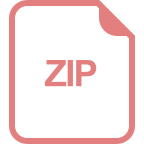
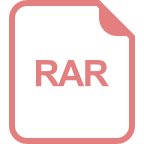
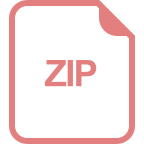
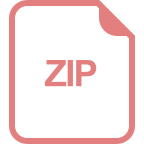
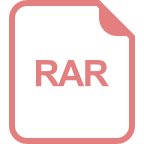
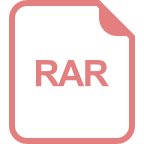
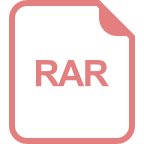
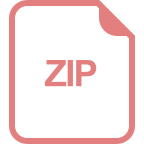