C++实现密码换位加密,输入输出明文,密文
时间: 2024-10-25 11:10:01 浏览: 27
在C++中,我们可以创建一个简单的密码换位加密算法,也称为凯撒密码(Caesar Cipher)。这种加密方法通过将字母表中的每个字符按照固定的偏移量向后移动来实现。这里是一个基础版本的实现示例:
```cpp
#include <iostream>
#include <string>
// 定义字符偏移量
const int OFFSET = 3; // 可以根据需要调整
std::string encrypt(const std::string& plaintext) {
std::string ciphertext;
for (char c : plaintext) {
if (isalpha(c)) { // 检查是否是字母
char shifted = static_cast<char>((c + OFFSET - 'A') % 26 + 'A'); // 遵循大写字母循环原则
ciphertext += shifted;
} else {
ciphertext += c; // 对非字母字符不做处理
}
}
return ciphertext;
}
std::string decrypt(const std::string& ciphertext, const int offset) {
return encrypt(ciphertext, -offset);
}
int main() {
std::string input = "Hello, World!";
std::string encrypted = encrypt(input);
std::cout << "Original text: " << input << "\n";
std::cout << "Encrypted text: " << encrypted << "\n";
std::string decrypted = decrypt(encrypted, OFFSET);
std::cout << "Decrypted text (using the same offset): " << decrypted << "\n";
return 0;
}
```
在这个例子中,`encrypt`函数接受明文字符串并返回密文,`decrypt`函数用于解密。在`main`函数中,我们展示了如何对输入的"Hello, World!"进行加密,并使用相同的偏移量进行解密。
如果你想让用户输入明文和偏移量,可以稍微修改上述代码添加用户交互部分。
阅读全文
相关推荐
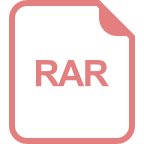
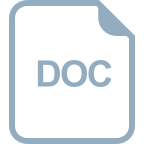
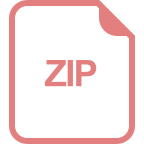

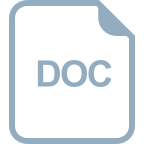
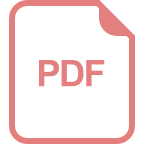
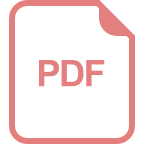
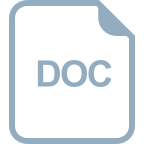
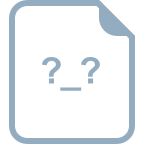
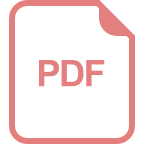
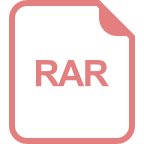
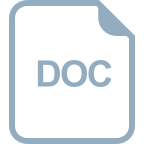
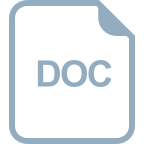
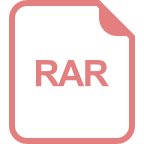
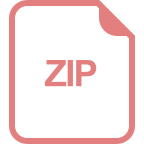
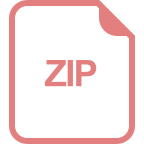
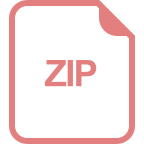