在线性表中插入元素c语言
时间: 2024-10-20 09:17:43 浏览: 17
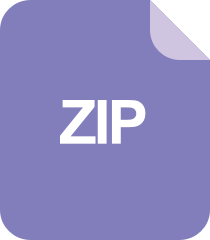
线性表综合题_C语言_线性表_
在线性表(如数组、链表等)中插入元素,在C语言中通常涉及以下几个步骤:
1. **数组插入**:
- 如果是在固定大小的数组中插入,一般需要移动后面的元素来空出位置,这涉及到数据的复制操作。
```c
void insert_array(int arr[], int size, int index, int element) {
// 检查索引是否合法
if (index < 0 || index > size) return;
// 将后面的元素向右移一位
for (int i = size; i > index; --i)
arr[i] = arr[i - 1];
// 插入新元素
arr[index] = element;
}
```
2. **动态数组(比如一维指针数组)插入**:
- 可能需要检查是否有足够的内存空间,然后动态分配。
```c
void insert_ptr_array(int** arr, int* capacity, int index, int element) {
// ...类似检查...
if (*capacity == MAX_SIZE) return; // 防止溢出
*arr = (int*)realloc(*arr, (*capacity + 1) * sizeof(int)); // 动态扩容
(*arr)[index] = element;
++(*capacity);
}
```
3. **链表插入**:
- 对于链表,直接在适当的位置创建一个新的节点并链接到现有节点。
```c
struct Node* insert_linkedList(struct Node* head, int value, int position) {
struct Node* newNode = malloc(sizeof(struct Node));
newNode->data = value;
newNode->next = NULL;
if (position == 0) { // 插入在头部
newNode->next = head;
head = newNode;
} else {
struct Node* current = head;
while (current->next != NULL && current->next->position < position - 1) {
current = current->next;
}
newNode->next = current->next;
current->next = newNode;
}
return head;
}
```
阅读全文
相关推荐
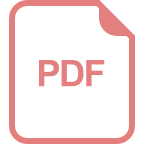
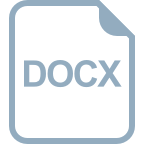
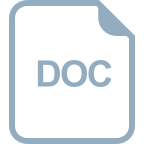
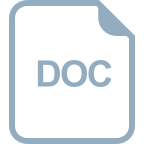
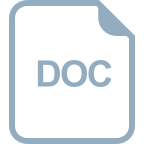





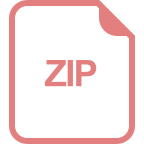
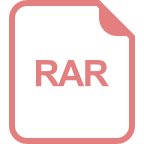
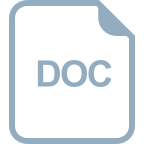




