在Tkinter中,pack()方法的after参数运用
时间: 2024-09-30 21:07:13 浏览: 31
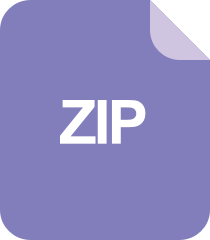
python 实现在tkinter中动态显示label图片的方法
在Tkinter中,`pack()`方法主要用于窗口部件的布局管理,而`after()`参数则是用来设置延时执行某些操作的功能。`after()`函数接受两个参数:延迟时间(以毫秒计)和一个回调函数或命令。
当你使用`after(interval, function)`时,它会在指定的时间间隔`interval`(例如500毫秒)过后,执行你传入的`function`。这个功能常用于创建定时任务、动画效果或者是实现一些需要定期更新的操作,比如每隔一段时间检查某个条件并作出响应。
例如,你可以这样使用:
```python
import tkinter as tk
from time import sleep
def check_and_update():
# 这里可以放置你的更新操作
print("Updating...")
sleep(1) # 模拟1秒后进行下一次检查
root = tk.Tk()
button = tk.Button(root, text="Click me")
button.pack()
# 设置按钮点击后的延时行为,每秒更新一次
button.after(1000, check_and_update)
root.mainloop()
```
在这个例子中,每次点击按钮后,`check_and_update`函数会被延迟1秒后执行。
阅读全文
相关推荐
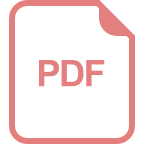
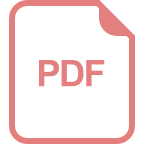
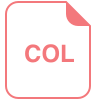
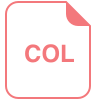













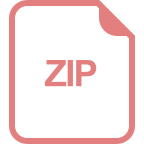