除了列表,还有其他方式创建二维数组吗?
时间: 2024-10-21 20:14:33 浏览: 25
除了列表之外,NumPy 还支持多种方式创建二维数组。例如:
1. **直接使用数值**:你可以直接提供一组有序数值给 `np.array`,系统会自动将其排列成一个二维数组。例如:
```python
array_2d = np.array([[1, 2], [3, 4]])
```
2. **numpy.meshgrid**:如果需要创建具有特定形状和坐标对的数组,可以使用 `meshgrid` 函数生成网格点,然后组合为二维数组:
```python
row = np.arange(3)
col = np.arange(4)
array_2d = np.array(np.meshgrid(row, col)).T.reshape(-1, 2)
```
3. **从文件读取**:如果你有包含二维数据的文本文件或二进制文件,可以用 `loadtxt` 或 `fromfile` 等函数读取并构造数组。
4. **从另一个数组复制**:通过切片或数组操作可以创建数组的副本,形成二维结构。
记得每种方式适用于不同场景,选择最适合你的需求的方法。
相关问题
在C语言中如何将一维数组输入二维数组中?
在C语言中,将一维数组赋值给二维数组通常涉及到数组指针和索引的操作。假设你有一个一维数组`one_dim_array[]`和一个二维数组`two_dim_array[row_size][col_size]`,你可以按行的方式逐个填充二维数组,示例代码如下:
```c
#include <stdio.h>
#define ROWS 3
#define COLS 4
void copyToTwoDim(int one_dim_array[], int two_dim_array[ROWS][COLS], int row, int col) {
for (int i = 0; i < sizeof(one_dim_array) / sizeof(one_dim_array[0]); i++) {
// 假设one_dim_array的元素数量正好等于两维数组的一行元素数量
two_dim_array[row][col++] = one_dim_array[i];
// 如果一行填满,移动到下一行
if (col == COLS) {
col = 0;
row++;
}
}
}
int main() {
int one_dim_array[ROWS * COLS]; // 创建一维数组,大小等于二维数组的元素总数
// ... 对一维数组进行初始化
int two_dim_array[ROWS][COLS]; // 初始化二维数组
copyToTwoDim(one_dim_array, two_dim_array, 0, 0); // 开始复制数据
// 输出或检查二维数组的内容...
return 0;
}
```
在这个例子中,`copyToTwoDim`函数接收一维数组、二维数组以及起始行和列作为参数,然后通过循环遍历一维数组,并将其元素插入到二维数组相应的位置。
如何动态创建二维数组?
在C++中,可以使用new操作符来动态创建二维数组。假设我们要创建一个m行n列的二维数组,可以使用以下语句:
```
int **array = new int*[m];
for(int i = 0; i < m; i++)
{
array[i] = new int[n];
}
```
以上代码会先创建一个指向int指针的指针array,然后使用循环分别为每一行分配n个int类型的内存空间。注意,在使用完这个二维数组之后,需要使用delete操作符来释放内存,否则会导致内存泄漏。
阅读全文
相关推荐
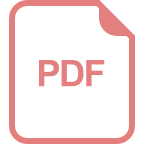
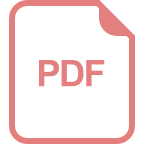
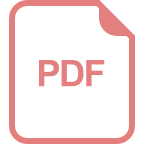

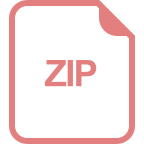
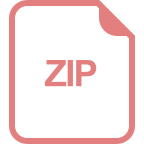









