编写C++程序,从标准输入分别读取两个整型操作数和一个运算符,整型操作数范围是0-2^32-1,运算符包括 + - * /,将每种运算实现为一个函数,计算过程中,通过try catch语句对加和乘运算进行溢出保护,对除法运算进行除零保护,并输出错误原因;
时间: 2024-09-10 10:07:47 浏览: 64
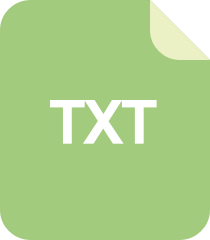
C++求两数之和
在C++中,你可以按照以下步骤编写这个程序:
```cpp
#include <iostream>
#include <limits>
// 定义运算函数模板,带异常处理
template <typename T>
T safe_arithmetic(T a, T b, char op) {
try {
switch (op) {
case '+':
return a + b;
case '-':
// 如果a小于b,可能会有负溢出
if (a > std::numeric_limits<T>::max() - b)
throw std::overflow_error("Integer overflow occurred.");
else
return a - b;
case '*':
// 如果乘积超过最大值,会抛出异常
if (std::numeric_limits<T>::max() / b < a)
throw std::overflow_error("Integer multiplication overflow occurred.");
else
return a * b;
case '/':
// 检查除数是否为零
if (b == 0)
throw std::runtime_error("Division by zero is not allowed.");
else
return a / b;
default:
throw std::invalid_argument("Invalid operator specified.");
}
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
return T(); // 返回默认值表示运算失败
}
}
int main() {
int a, b;
char op;
// 从标准输入读取
std::cout << "Enter two integers and an operator (+, -, *, /): ";
std::cin >> a >> b >> op;
// 调用安全运算函数并打印结果
int result = safe_arithmetic(a, b, op);
if (result != T()) { // 如果没有异常,打印结果
std::cout << "Result: " << result << std::endl;
}
return 0;
}
```
在这个程序中,`safe_arithmetic`函数是一个通用模板函数,接受两个操作数和一个运算符作为参数,然后根据运算符执行相应的操作。如果遇到溢出或除零等错误,会捕获异常并输出错误信息。
在`main`函数中,用户会被提示输入两个整数和一个运算符,然后调用`safe_arithmetic`函数并处理其返回结果。
阅读全文
相关推荐
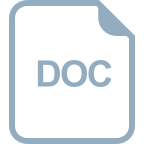
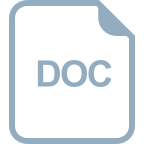
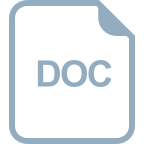
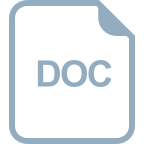
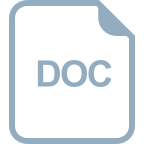
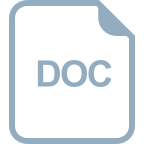





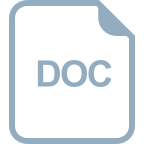
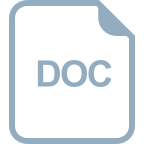
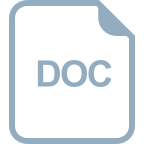
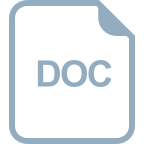
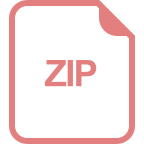
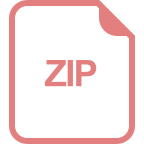
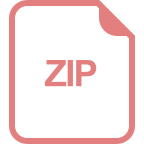