运用所学到的知识,编写一个名为dcalendar.c的程序,打印今年某一周的周历l提示:先求出该周的起始日、结束日是全年的第几天,以全年第几天为循环变量打印周历,循环体中判断所打印日期是几月几号
时间: 2024-10-17 08:15:12 浏览: 10
为了编写一个名为`dcalendar.c`的程序,我们首先需要了解一些基本的编程概念,比如获取当前日期、计算日期之间的差值、以及使用条件语句。以下是一个简单的C程序示例,它使用`<time.h>`库来获取当前日期并计算指定周的日期范围:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
// 定义结构体表示日期
struct Date {
int day;
int month;
};
// 获取当前日期
struct Date get_current_date() {
time_t current_time = time(NULL);
struct tm *time_info = localtime(¤t_time);
return (struct Date) {time_info->tm_mday, time_info->tm_mon + 1};
}
// 计算指定周的起始和结束日期
struct Date calculate_week_range(struct Date start_date, int week_start_day) {
int days_per_month[] = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31}; // 简化版本,未考虑闰年
int total_days = start_date.day + sum_days(start_date.month - 1, days_per_month);
// 起始日期加(week_start_day - 1)
struct Date week_start = {start_date.day + (week_start_day - 1), start_date.month};
// 结束日期为本周最后一天(假设一周七天)
struct Date week_end = {week_start.day + 7, week_start.month};
// 调整闰年的2月份天数
if (is_leap_year(week_start.year) && week_start.month == 2) {
week_end.day += 1;
}
return week_start;
}
// 判断是否为闰年
int is_leap_year(int year) {
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
// 计算前n个月的总天数
int sum_days(int n, int days[]) {
int total = 0;
for (int i = 0; i <= n; i++) {
total += days[i];
}
return total;
}
// 打印周历
void print_week_calendar(int year, int week_start_day) {
struct Date start_date = {1, 1}; // 假设我们要从新年第一天开始
struct Date range = calculate_week_range(start_date, week_start_day);
for (struct Date date = range; date.day <= range.day + 6; date.day++) {
printf("%02d/%02d\n", date.day, date.month);
}
}
int main() {
int current_year = 2023; // 使用当前年份
int week_start_day = 1; // 我们假设一周的第一天是从星期一开始
print_week_calendar(current_year, week_start_day);
return 0;
}
```
这个程序首先获取当前日期,然后计算给定的一周起始和结束日期。接下来,它通过循环打印这一周的所有日期。注意,这是一个简化版本,实际的`dcalendar.c`可能会更复杂,包括处理闰年、输入用户选择的日期和周数等。
阅读全文
相关推荐
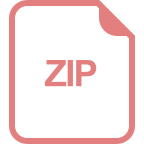
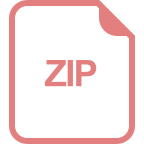
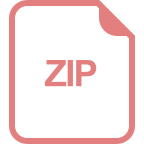















