用java语言实现银行家算法
时间: 2024-05-13 16:11:58 浏览: 102
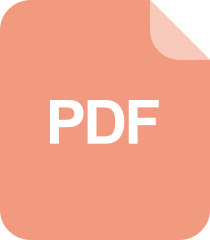
使用java实现银行家算法

银行家算法是用于避免死锁的一种算法。在多进程并发的环境下,如果进程请求的资源超过了系统所拥有的资源,就可能会导致死锁的情况出现。银行家算法可以通过合理地分配资源来避免死锁的出现。
Java语言实现银行家算法可以参考以下步骤:
1. 定义资源类(Resource),包括资源名称、资源数量、资源状态等属性;
2. 定义进程类(Process),包括进程名称、进程需要的资源数量、进程已经分配到的资源数量、进程状态等属性;
3. 定义银行家类(Banker),包括系统当前拥有的资源数量、系统当前可用的资源数量、已经分配给进程的资源数量等属性,以及分别处理进程请求资源和进程释放资源的方法;
4. 在银行家类中实现银行家算法,处理进程请求资源时检查系统当前是否能够满足进程的请求,如果能够满足就分配资源并更新系统状态,否则暂时不分配资源并将该进程加入等待队列中;
5. 在银行家类中实现进程释放资源的方法,当一个进程释放资源时,将已经释放的资源加回到系统可用资源中,并更新系统状态;
6. 根据需要在主函数中实例化资源、进程和银行家对象,模拟进程请求和释放资源的情况,检查系统状态是否正常。
阅读全文
相关推荐
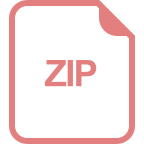
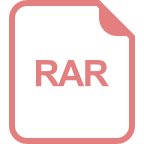
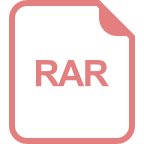
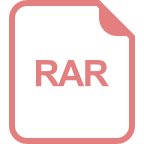
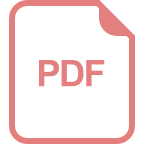
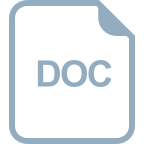
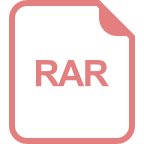
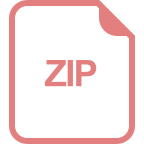
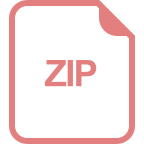
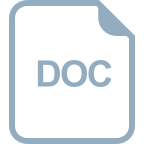
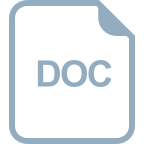



